Anonymize Calls Between Users
Using number masking to set up a scenario where two or more parties can call each other anonymously. A common scenario when you need to set this up is with in-app calls in ridesharing or in ecommerce, for example, where you want to enable drivers to call the user without knowing their real number.
There are multiple ways to implement this using the Infobip platform and Voice & Video as a channel. This tutorial shows you how to implement a simple in-app calling feature in your online application.
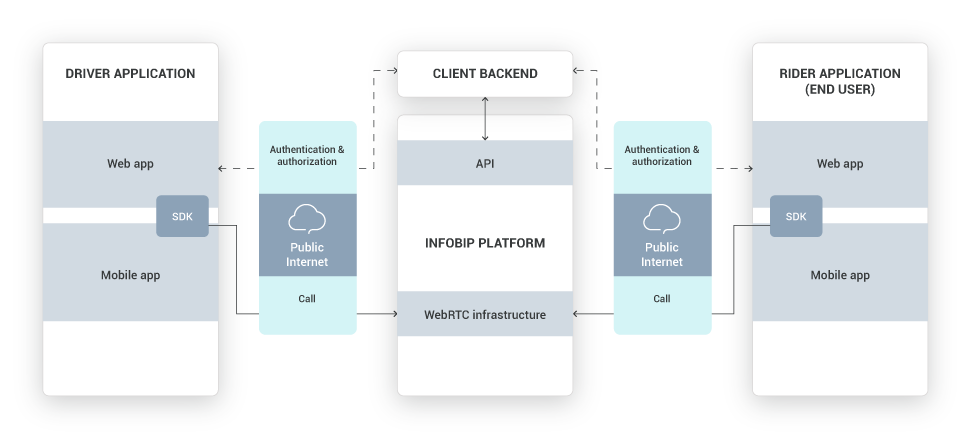
Process Workflow
User Registration Flow
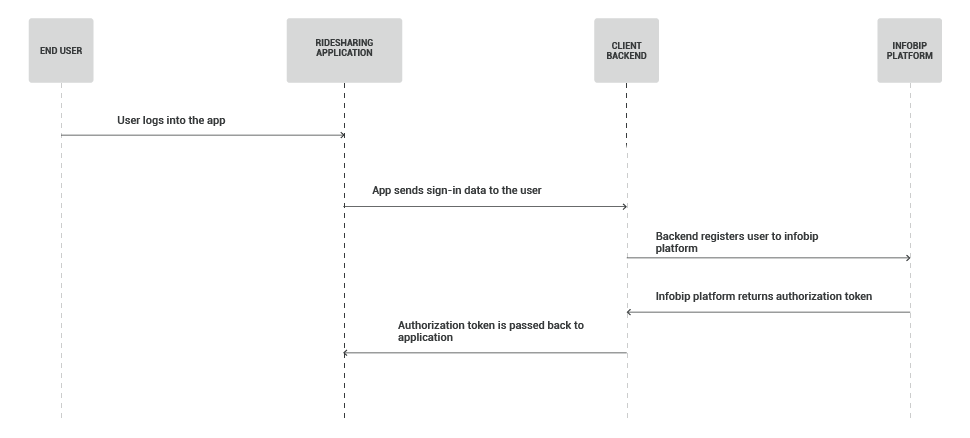
Call Flow
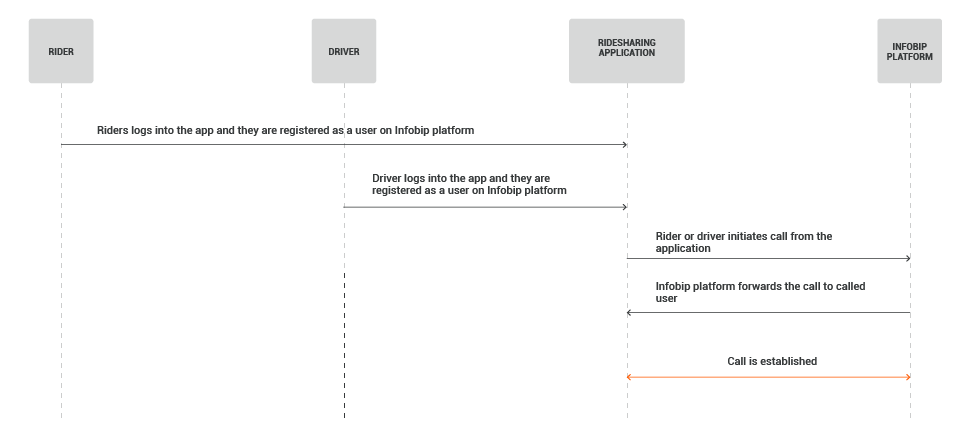
IMPLEMENTATION STEPS
Steps over SDK
To use Infobip RTC, you need to have Web and In-App Calls enabled on your account.
-
First and foremost, include Infobip RTC SDK(s) in your application(s):
-
Register your users (opens in a new tab) on the Infobip platform over API. Users are registered with their identity – a unique alphanumeric string. Once completed, the Infobip platform will return an authorization token.
Request example:
jsonPOST /webrtc/1/token HTTP/1.1 Host: https://www.infobip.com/docs Authorization: Basic QWxhZGRpbjpvcGVuIHNlc2FtZQ== Content-Type: application/json Accept: application/json {"identity":"Alice","displayName":"Alice in Wonderland" }
Response example:
json{ "token": "3981e92d-141e-48bb-8e10-f751e0b4bfb5", "expirationTime": "2019-02-06T11:10:00.123Z" }
-
Initiate a call from one registered user towards the other registered user via the call method. Authorization token needs to be supplied that you will have received from the previous response.
Example:
swiftimport Foundation import InfobipRTCclass Foo { func makeCall() { let token = obtainToken() let callRequest = CallRequest(token, destination: "Alice", callDelegate: self) do { let outgoingCall = try InfobipRTC.call(callRequest) } catch CallError.callInProgress { print("Failed to make a call, already running active call.") } catch CallError.invalidDestination { print("Failed to make call, invalid destination.") } catch CallError.noInternetConnection { print("Failed to make call, no internet connection.") } catch CallError.missingMicrophonePermission { print("Failed to make call, missing microphone permission.") } catch { print("Failed to make call") } } }extension Foo: CallDelegate { func onEstablished(_ callEstablishedEvent: CallEstablishedEvent) { print("Call with Alice established.") } func onHangup(_ callHangupEvent: CallHangupEvent) { print("Call with Alice finished.") } func onError(_ callErrorEvent: CallErrorEvent) {} func onEarlyMedia(_ callEarlyMediaEvent: CallEarlyMediaEvent) {} func onRinging(_ callRingingEvent: CallRingingEvent) {} }
swiftfunc pushRegistry(_ registry: PKPushRegistry, didReceiveIncomingPushWith payload: PKPushPayload, for type: PKPushType) { if type == .voIP { if var incomingCall = InfobipRTC.handleIncomingCall(payload) { incomingCall.delegate = self incomingCall.accept() // or incomingCall.decline() } } }
PRO TIPIf the called user is currently offline and the call cannot be established over the internet then you can implement failover calls to the GSM network. Initiate a call towards a landline or mobile phone by using the callPhoneNumber method.
Example:
swiftlet token = obtainToken() let callRequest = CallRequest(token, destination: "41793026727", callDelegate: self) do { let outgoingCall = try InfobipRTC.callPhoneNumber(callRequest, CallPhoneNumberOptions(from: "33755531044")) } catch { print("Failed to make phone call.") }
PRO TIPTake a look at our Dynamic Destination Resolving for a full integration of phone and VoIP calls.
PRO TIPIn case you are interested in phone calls only, then Infobip Number Masking solution might be the right solution for you.