SMS over API
Learn how to receive SMS API and to send SMS message API requests.
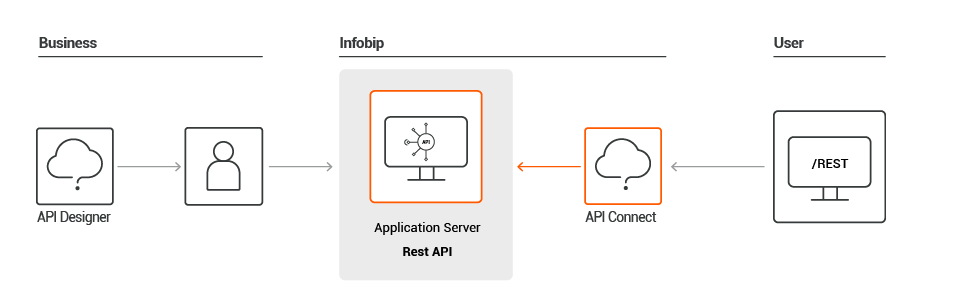
Basic API for sending SMS
If you want to automatize your SMS traffic, and you don’t want to use our SaaS solutions like Flow, you can always use our API. If you don't need a robust solution, use our most basic API request which only requires three parameters to send a message.
Request
POST /sms/2/text/advanced
{
"messages": [
{
"from": "InfoSMS",
"destinations": [
{
"to": "41793026727"
}
],
"text": "This is a sample message"
}
]
}
For more details, visit our SMS API documentation (opens in a new tab).
Fully Featured API for sending SMS
If you need advanced functionalities like validity period, transliteration, etc., use our fully featured API (opens in a new tab). You can also use the version of our API where parameters can be set directly in the URL.
To receive an SMS message, or to transfer received SMS messages from our platform to yours, you can:
- PULL messages you received to your number. These messages are stored on our platform and you can pull them when it suits you best. Refer to the Get received messages (opens in a new tab) for more details.
- PUSH received messages automatically to your dedicated URL.
Both of these of methods require you to set up a number and a type of forwarding actions for it, so that our platform knows what to do.
If you haven't set up forwarding to a specific number, check your inbound logs for any received messages. This is very similar to our PULL action, however, logs only store your data for up to 48 hours.
SMS Preview
To avoid unpleasant surprises, use this method (opens in a new tab) to preview messages before sending them out to your customers. Discover the message length, a number of message parts, language, and transliteration output, etc.
The preview method can provide you with the following information:
- originalText- original message content that was supplied for preview
- textPreview- preview of the message content as it should appear on the recipient's device
- messageCount- number of messages required to deliver textPreview
- charactersRemaining- number of remaining characters before messageCount increase
- configuration- configuration setup that should be included in advanced SMS in order to deliver textPreview message
- languageCode- language code for the correct character set
- transliteration- the transliteration of your sent message from one script to another; used to replace characters which are not recognized as part of your defaulted alphabet.
Message Count and Remaining Characters
Use this method to see how many characters are left ‘unspent’ in the message and if the message fits in one or more messages.
Request
POST /sms/1/preview
{
"text": "Let's see how many characters will remain unused in this message."
}
Response
{
"originalText": "Let's see how many characters will remain unused in this message.",
"previews": [
{
"textPreview": "Let's see how many characters will remain unused in this message.",
"messageCount": 1,
"charactersRemaining": 95,
"configuration": {}
}
]
}
The response contains the original text (sent text) and one preview which showcases a message received by the recipient without any configuration. In this case, the text will fit in one message and there will be 95 characters left. No special configuration is needed if your goal is to deliver the message as seen in textPreview.
Notify URL
Use the Notify URL feature to forward Delivery Reports, message status, pricing information or GSM errors (e.g. EC_ABSENT_SUBSCRIBER) to your callback server using a predefined URL. If set up, Notify URL forwards the data as soon as the Infobip platform receives it. Use the "notifyUrl" field to specify the URL.
In addition to the URL, you can also specify the following fields to make the feature more robust:
- notifyContentType- determines which body type to push to the server, JSON or XML.
- messageId- customize your message ID, so that your response contains the same ID and is easier to find; otherwise, Infobip will automatically generate it for you in a response.
- bulkId- customize your bulk ID if sending multiple messages or to multiple recipients, so that your response contains the same ID and is easier to find; otherwise, Infobip will automatically generate it for you in a response.
- callbackData- additional user-defined data that will be sent on to the Notify URL.
Request
POST /sms/2/text/advanced
{
"bulkId": "june-campaign",
"messages": [
{
"from": "SomeStore",
"destinations": [
{
"to": "41793026727",
"messageId": "silver-loyalty-program"
},
{
"to": "41793026731",
"messageId": "golden-loyalty-program"
}
],
"text": "Check out our newest collection available NOW in your local store.",
"notifyUrl": "http://www.example.com/sms/campaigns",
"notifyContentType": "application/json",
"callbackData": "For example, this field can store tracking ID for analytics"
}
]
}
Push Retry Cycle
If your Notify URL is unavailable for any reason, forward attempts will be made according to formula:
1min + (1min * retryNumber * retryNumber)
Examples for first few retry attempts are shown in the table below. Maximum number of retries is 20, i.e. the last retry will be done 41:30h after the initial one. If your URL is not available for the whole retry period, the data will be lost.
Retry | Interval | Cumulative |
---|---|---|
0 | 01min | 00:01h |
1 | 02min | 00:03h |
2 | 05min | 00:08h |
3 | 10min | 00:18h |
4 | 17min | 00:35h |
5 | 26min | 01:01h |
6 | 37min | 01:38h |
SMS to Multiple Destinations
Imagine you have opened a wine shop and you want to invite your future customers for the grand opening. Do it over SMS—one of the most powerful promotional channels!
You can send the same message to multiple phone numbers. The example below only contains two phone numbers for easier understanding.
Request
POST sms/2/text/advanced
{
"messages": [
{
"from": "WineShop",
"destinations": [
{
"to": "41793026727"
},
{
"to": "41793026727"
}
],
"text": "Wine shop grand opening at Monday 8pm. Don't forget your wine glass!"
}
]
}
Here's the three parameters you should take a closer look into:
- from - represents the sender of the SMS message - it can be alphanumeric or numeric. Alphanumeric sender ID length should be between 3 and 11 characters (Example: CompanyName). Numeric sender ID length should be between 3 and 16 characters.
- to - an array of message destination addresses. Destination addresses must be in international format (e.g. 41793026727).
- text - content of the message being sent.
This will send an SMS to two addresses with the same content and sender. The response will also contain a "bulkId" field to group your multiple recipients under one response so that you can easily fetch logs or reports. You can pass your custom Bulk ID in the request, otherwise if omitted, the Infobip platform will automatically generate one and assign it to your response.
Response
{
"bulkId": "2034070510160109025",
"messages": [
{
"to": "41793026727",
"status": {
"groupId": 1,
"groupName": "PENDING",
"id": 26,
"name": "PENDING_ACCEPTED",
"description": "Message sent to next instance"
},
"messageId": "2034070510160109026"
},
{
"to": "41793026834",
"status": {
"groupId": 1,
"groupName": "PENDING",
"id": 26,
"name": "PENDING_ACCEPTED",
"description": "Message sent to next instance"
},
"messageId": "2034070510250109027"
}
]
}
Your response will include the following:
- bulkId - used for getting delivery reports (opens in a new tab) for SMS messages sent to multiple destinations.
- to - the recipient of the message
- status - indicates the message status (opens in a new tab)
- smsCount - represents the number of SMS messages sent to one destination
- messageId - uniquely identifies the message sent
Multiple SMS to Multiple Destinations
Imagine you want to target your customers by offering them their favorite product during your next promotion. Every customer is specific, and you shouldn’t send the same message to all of them. Tailor your SMS messages according to customers’ preferences and get their full attention.
You can use our multiple textual messages for that purpose. This option gives you the ability to send multiple messages to multiple destinations by calling one API method only once.
Request
POST /sms/2/text/advanced
{
"messages": [
{
"from": "WineShop",
"destinations": [
{
"to": "41793026727"
}
],
"text": "Hey Mike, delicious Istrian Malvazija is finally here. Feel free to visit us and try it for free!"
},
{
"from": "WineShop",
"destinations": [
{
"to": "41793026834"
}
],
"text": "Hi Jenny, we have new French Merlot on our shelves. Drop by our store for a free degustation!"
}
]
}
After you have sent these messages, you will be able to get detailed stats and analyze them. For example, you can measure how many customers received the SMS invitation by getting the delivery reports (opens in a new tab).
Refer to Response Status and Error Codes for further information on your message status.
Send SMS message over query parameters
Send SMS by including all of the required URL parameters in one query string. This is a perfect solution if you have an application with limited integration possibilities and you are not able to fully integrate with the REST API method for sending an SMS (opens in a new tab).
Request
curl -L -g -X GET 'https://{baseUrl}/sms/1/text/query?username={username}&password={password}&from=InfoSMS&to=41793026727,41793026834&text=Message%20text&flash=true&transliteration=TURKISH&languageCode=TR&intermediateReport=true¬ifyUrl=https://www.example.com¬ifyContentType=application/json&callbackData=callbackData&validityPeriod=720&track=URL&trackingType=Custom%20tracking%20type' \
-H 'Authorization: {authorization}' \
-H 'Accept: application/json'
This is all you need to send a message. You can even send this message through your web browser address bar, the same way you would type an address to open a web page. This is something that you could put into your application without writing any code.
Response
The response will is identical to a fully-featured SMS response.
{
"bulkId": "1478260834465349757",
"messages": [
{
"to": "41793026727",
"status": {
"groupId": 1,
"groupName": "PENDING",
"id": 7,
"name": "PENDING_ENROUTE",
"description": "Message sent to next instance"
},
"smsCount": 1,
"messageId": "844acc75-e5c6-4a21-a7e3-444c412c385b"
}
]
}
The default response format is JSON. However, if you would like to receive XML instead, you may specify the format .xml
extension at the end of the method URL, such as: https://api.infobip.com/sms/1/text/query.xml
.
You need to replace hard-coded values like phone number and message text with placeholders, unless you want to send the same message to the same recipient every time. Placeholder names are provided by the application system you are using. All you need to understand is where to put them.
Using the example, this breaks down the request in several lines to examine it more closely:
https://api.infobip.com/sms/1/text/query?
username=myUsername
&password=myPassword
&to=41793026727
&text=Message text
The first line is the API endpoint and this never changes. The question mark at the end represents the beginning of the query string parameters. This is a system of key /value pairs separated by symbol &
. This enables you to see what the values are and which values you need to replace by a value placeholder. For example:
&to=%phoneNumber%
&text=%message%
Placeholder names and format will vary depending on your application. See your application documentation for instructions.
This is the final result:
https://api.infobip.com/sms/1/text/query?username=myUsername&password=myPassword&to=%phoneNumber%&text=%message
Your application should now dynamically send different messages to different recipients and dynamically replace placeholders with the recipient’s phone number and message.
Track Conversion
Conversion tracking involves monitoring and analyzing marketing campaign performance to understand how well your message resonates with its target audience.
SMS conversion tracking allows you to notify the Infobip platform about a successful conversion of specific user actions. Typical user actions include, clicks on URL clicks, code input, form submissions, purchases, or other desired outcomes after receiving an SMS.
Once an action has taken place, information is sent to the Infobip platform for performance analysis.
Why is conversion tracking important
Conversion tracking helps Infobip to see a better picture of how your traffic is performing and proactively take measures to correct any degradation in quality.
Providing detailed insights into customer behavior and campaign performance enables businesses to refine their marketing strategies, improve customer engagement, and ultimately drive more sales and revenue. Implementing SMS conversion tracking can significantly enhance the effectiveness of business marketing campaigns.
You can submit conversion information only for SMS messages. The goal of conversion tracking is to keep track of time-sensitive messages, like 2FA passwords, or messages that expect a quick reaction from your end users, like clicking a specific link.
Send SMS with conversion tracking
Enable tracking by setting up a conversionTracking
object within your message body.
For more details on how to send an SMS with conversion tracking enabled, see the API Reference (opens in a new tab).
A messageId
is returned in the POST Send SMS Message response, which you need to provide to Infobip, as follows:
- The Infobip platform marks the start of the tracking process as soon as the message is sent out.
- Once the message is delivered and an end user performs an action (clicks a link, inputs a code, and so on), this counts as a successful conversion rate. You can track such actions through webhooks implemented on your side.
- Notify Infobip about the successful conversion by calling
POST /ct/1/log/end/{messageId}
.
Once the Infobip platform is notified about the successful conversion, it can monitor the performance and use this data to provide you with a better service.