Code in Coding Element
This section provides examples of how to use the Code element to perform various tasks.
Transfer end-user's location into latitude and longitude
When the end user sends the location and you want to determine exact latitude and longitude.
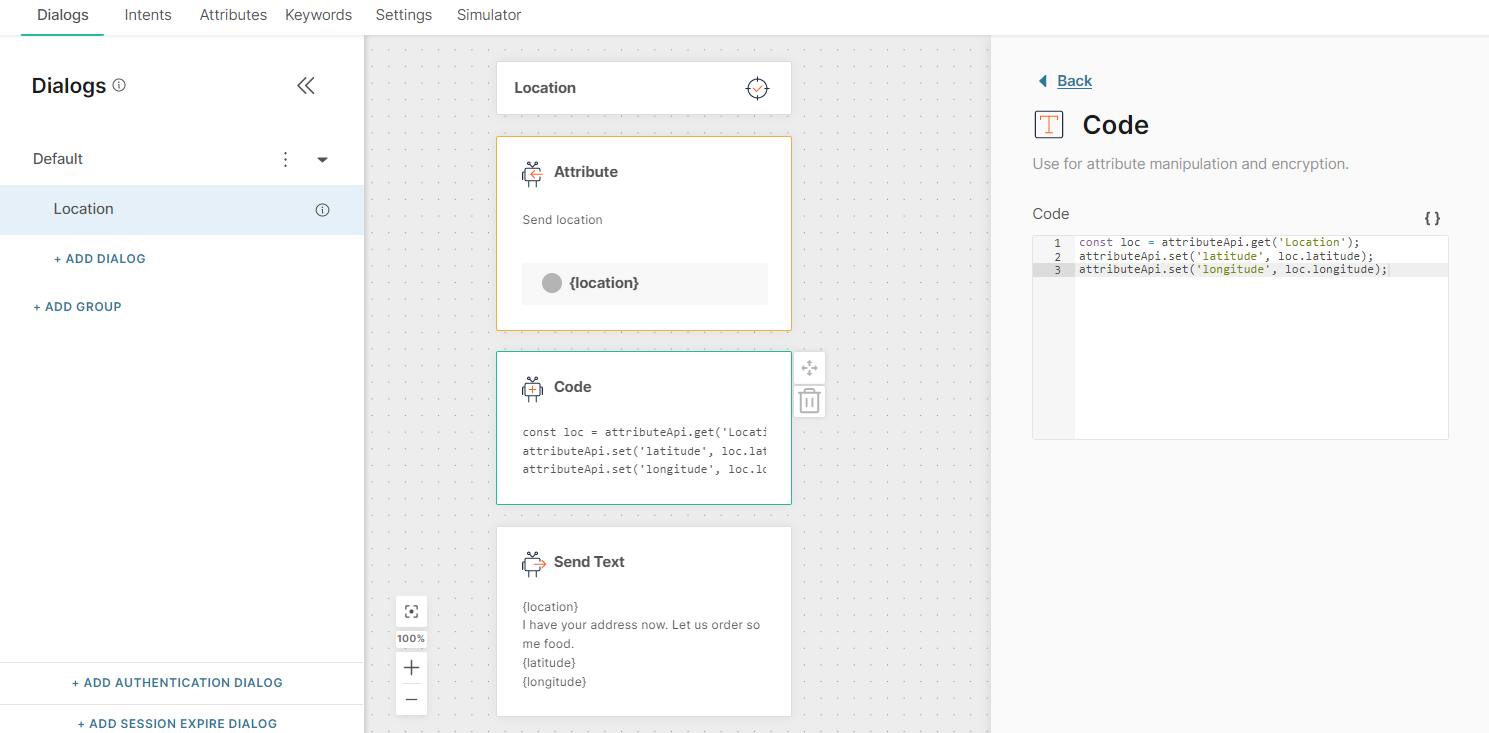
const loc = attributeApi.get('Location');
attributeApi.set('latitude', loc.latitude);
attributeApi.set('longitude', loc.longitude);
Define working hours
If the end user wants to talk to an agent, they can reach them during working hours. But outside of working hours, the end user won't be redirected to an agent.
With the coding element, you can define those working hours and configure the dialog accordingly.
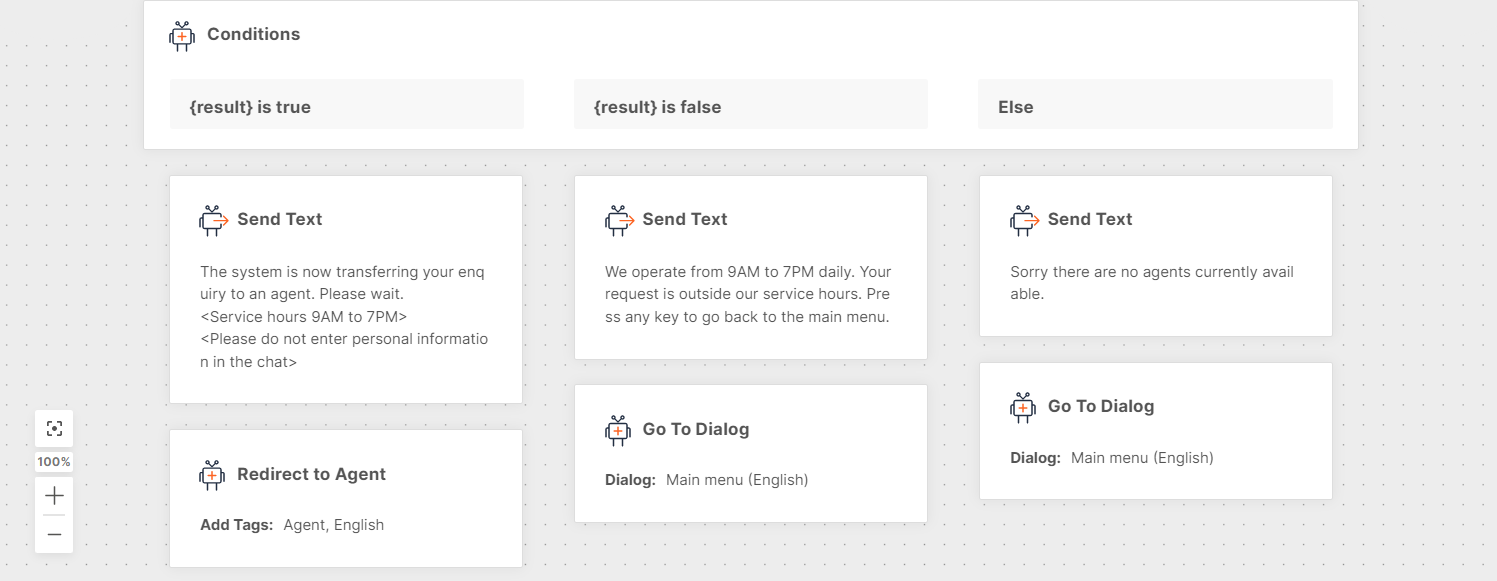
Take into account the different time zones (opens in a new tab).
const Monday = { open: 8, close: 17 };
const Tuesday = { open: 8, close: 17 };
const Wednesday = { open: 8, close: 17 };
const Thursday = { open: 8, close: 17 };
const Friday = { open: 8, close: 17 };
const Saturday = { open: 0, close: 0 };
const Sunday = { open: 0, close: 0 };
const myTimeZone = 'Europe/Zagreb'; // This IANA time zone name
const workingHoursSchedule = [Sunday, Monday, Tuesday, Wednesday, Thursday, Friday, Saturday];
function isWorkingHours(now) {
const day = new Date(now).getDay();
const hours = new Date(now).getHours();
const daySchedule = workingHoursSchedule[day];
return hours >= daySchedule.open && hours < daySchedule.close;
}
const result = isWorkingHours(new Date().toLocaleString('en-US', {
timeZone: myTimeZone
}));
attributeApi.set('result', result);
Branch dialogs according to incoming promo messages
Use lastreceivedtextmessage predefined attribute to check whether incoming messages contain a promo code.
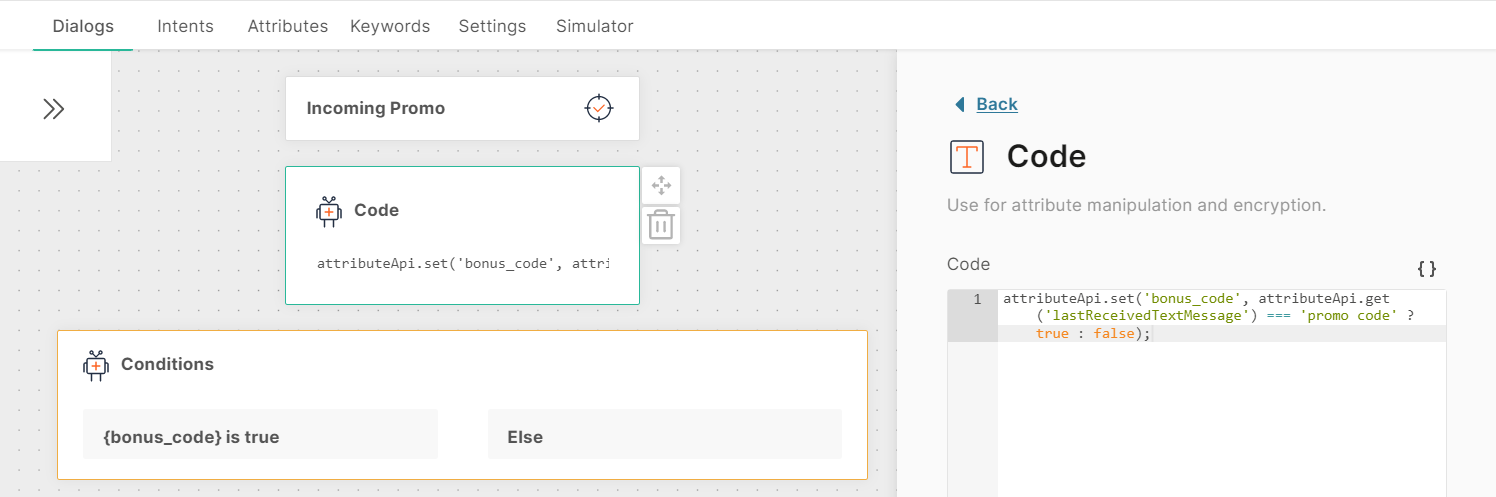
attributeApi.set('bonus_code', attributeApi.get('lastReceivedTextMessage') === 'promo code' ? true : false);
Initialize parameters only once using Global Attributes
In many instances when creating a chatbot, you need to make multiple API calls for various use cases that the bot supports. Instead of hardcoding the API details such as the base URL, username, password, encryption key (if applicable), etc., you can create global attributes for each of these parameters and then initialize them using the coding element once the user enters the default dialog.
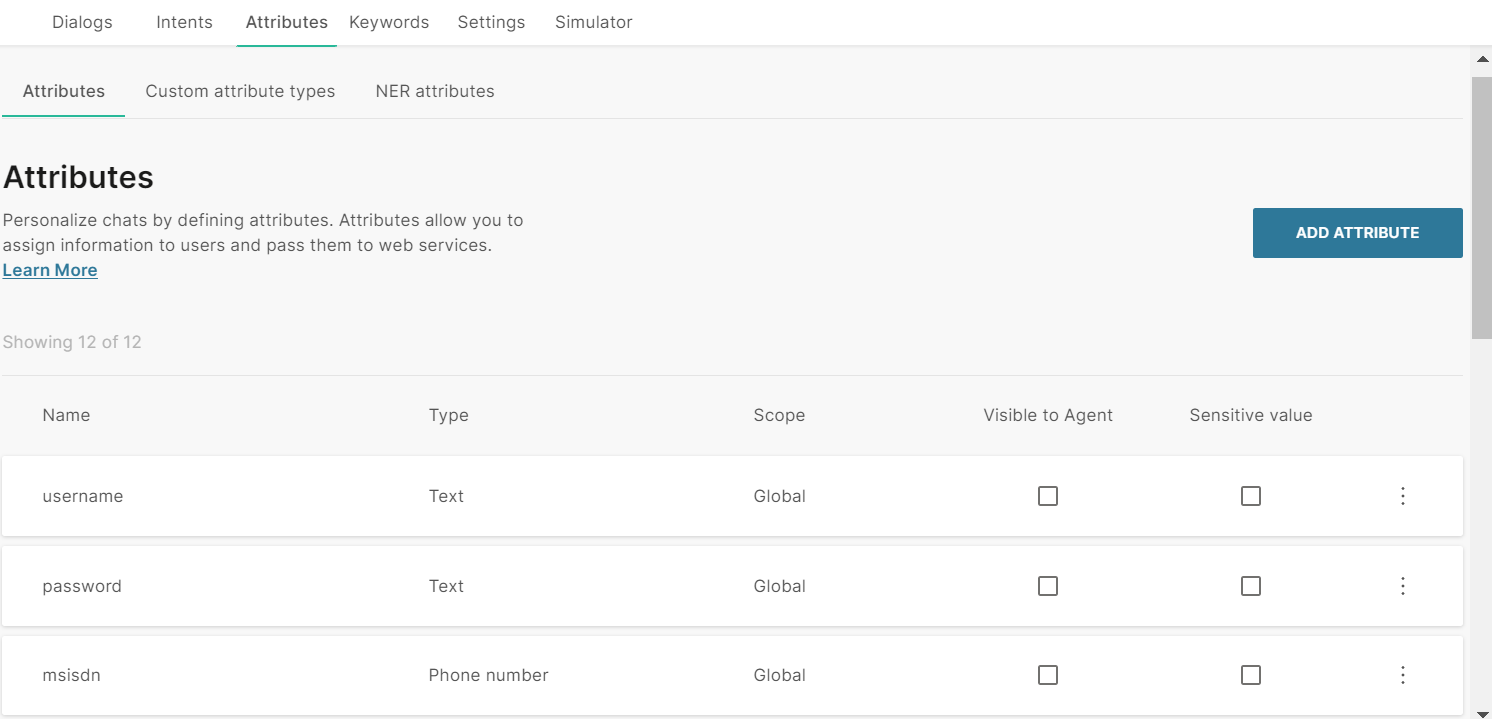
This is the code you can use for initializing values:
attributeApi.set('base_url', 'https://127.0.0.1:9030');
attributeApi.set('msisdn', attributeApi.get('phoneNumber'));
attributeApi.set('username', 'someUsername');
attributeApi.set('password', 'somePassword');
// or
attributeApi.set('password', encryptionUtils.sha1('somePassword'));
The initialized values are then accessible in all dialogs in the chatbot as attributes (without hard coding them everywhere you need to use them).
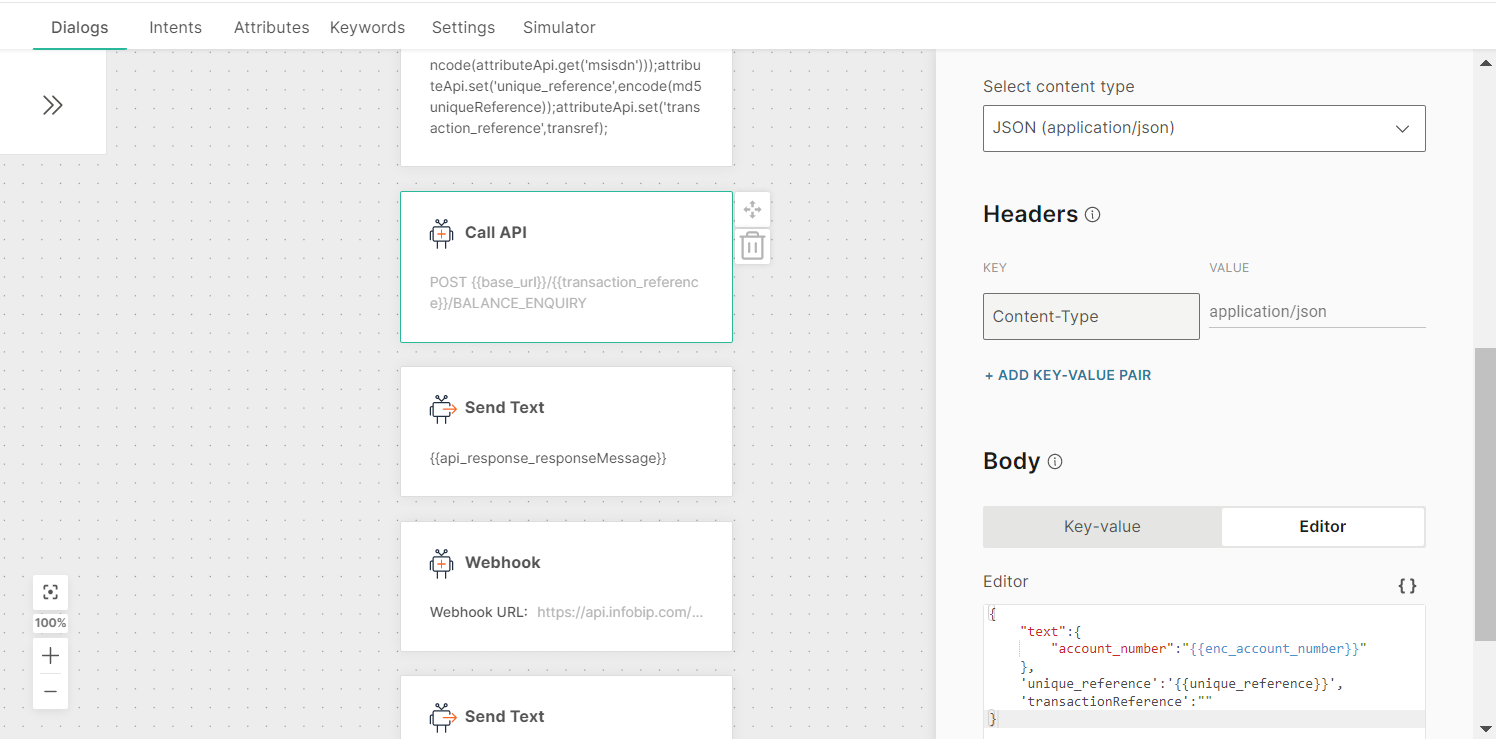
Should you need to change the bot environment from development to production, and the API URL and credentials change, you just need to change those details only once in the coding element.
Compare numerical attribute values
For example, if you have customers applying for loans, and the customer should not be allowed to apply for a loan amount greater than their credit score (loan limit). This loan limit varies from customer to customer and cannot be hardcoded.
-
Create a global Boolean attribute is_valid_loan_amount
-
After the user enters loan amount to borrow (loan_amount), use the coding element to compare the loan amount Vs the loan limit (credit_score), setting the is_valid_loan_amount to true or false depending on the comparison results
javascriptconst creditScore = attributeApi.get('credit_score'); const loanAmount = attributeApi.get('loan_amount'); if (loanAmount <= creditScore) { attributeApi.set('is_valid_loan_amount', true); } else { attributeApi.set('is_valid_loan_amount', false); }
-
Add a Conditions element, such that if is_valid_loan_amount is true, proceed with loan application, else notify the user to apply for a lesser amount.
Select a valid option from dynamically generated API response
For example, if you have a banking chatbot, and the end user is presented with a list of account numbers to transact from, they should select one account number from the list. If the selected account number is not in the provided list then the bot should respond with an error message.
-
Create a list attribute response_accounts to store the list of user bank accounts from the API response. Define the account_number attribute which will hold the account number the user has typed in.
-
Display the dynamic list of accounts for the user to select from. The user input will be stored in the account_number attribute.
-
Using the coding element, validate whether the user input (account_number) is in the list of the valid account numbers:
javascriptconst validAccounts = attributeApi.get('valid_accounts'); const userInput = attributeApi.get('account_number'); let accountNumber = null; const isUserInputValidAccount = validAccounts.indexOf(userInput) > -1; if (isUserInputValidAccount) { accountNumber = userInput; } else { const indexInArray = userInput - 1; const isUserInputValidArrayIndex = validAccounts.length > indexInArray && validAccounts[indexInArray] !== 'undefined'; if (isUserInputValidArrayIndex) { accountNumber = validAccounts[indexInArray]; } } attributeApi.set('account_number', accountNumber);
-
In the above coding element, if the account_number is not valid (i.e. it’s not in the valid list), its set to null.
-
Using the conditions element, if the account_number attribute is null, display the invalid account number message, else continue conversing with the bot:
Allow multiple selection from a dynamically generated API response
When you have an onboarding chatbot, and want the end users to select their disability, it can be selected from a dynamically generated list (using API call). Each disability has an ID and a Name. To enable users to select multiple values, they can type in several numbers separated by space. Using a second API, the selected disability IDs (not the number options the user typed in) are passed as a single white-space delimited parameter.
-
Create the following attributes:
disabilities_response – will hold the list of disabilities to select from (returned by the first API)
user_selection – Will hold the list of disabilities the user has selected
disability_found –a Boolean attribute keeping track whether the user input is valid
user_input – will store the disabilities that the user types in
api_response – complete api response in the root json path($.)
invalid_input –the user input that doesn’t match expected responses
selected_ids – the disability IDs that the user selected
-
Display the dynamic list of disabilities for the user to select. The user input will be stored in the user_input attribute. This user input is validated using a regex expression that only allows numbers and spaces (^[0-9\s]*$)
-
Using the coding element:
a) Convert the space delimited user input into an array for easier processing
b) Loop through the elements in the array generated in the previous step. For each iteration, check if the current element (user input) is an index in the list of disabilities. If it is, store the corresponding disability in the user_selection list attribute defined in step 1. For good measure, you can separately push the matched disability ID to a separate list selected_ids (also defined in step 1).
c) Convert the selected_ids list into a space delimited string as expected by the second API call.
The final coding element should be like this:javascript/** * This validates disability type based on user input **/ var user_input = attributeApi.get('user_input'); var disabilities = attributeApi.get('disabilities_response'); var inputs_array = user_input.split(" "); var selected_ids = []; attributeApi.set('inputs_array', inputs_array); var user_selection = []; var disability_found = true; var i = 0; for(i=0; i<inputs_array.length; i++){ var val = inputs_array[i]; var index = parseInt(val)-1; attributeApi.set('invalid_input', val); if(val == "") {//Means multiple white spaces between numbers //Pass }else if(typeof disabilities[index] == 'undefined') { disability_found = false; attributeApi.set('invalid_input', val); } else { //Found user_selection.push(disabilities[index]); selected_ids.push(disabilities[index].Id); } } attributeApi.set('disability_found', disability_found); attributeApi.set('user_selection', user_selection); attributeApi.set('selected_ids', selected_ids.join(" "));
-
Using the conditions element, if disability_found attribute is True, proceed with chatbot conversation, otherwise display a validation error message.
Transfer time and date sent by the user into required time zone and date format
In the example below, the time zone to which the time and date received are reformatted in the Coding element is Asia/Kolkata:
const date = new Date();
const year = new Intl.DateTimeFormat('en', { timeZone: 'Asia/Kolkata', year: 'numeric' }).format(date);
const month = new Intl.DateTimeFormat('en', { timeZone: 'Asia/Kolkata', month: '2-digit' }).format(date);
const day = new Intl.DateTimeFormat('en', { timeZone: 'Asia/Kolkata', day: '2-digit' }).format(date);
const hour = new Intl.DateTimeFormat('en', { timeZone: 'Asia/Kolkata', hour: '2-digit', hour12: false }).format(date);
const minute = new Intl.DateTimeFormat('en', { timeZone: 'Asia/Kolkata', minute: '2-digit' }).format(date);
const second = new Intl.DateTimeFormat('en', { timeZone: 'Asia/Kolkata', second: '2-digit' }).format(date);
const milli = date.getMilliseconds();
const formattedDate = `${year}-${month}-${day} ${hour}:${minute}:${second}.${milli}`;
attributeApi.set('Date', formattedDate);
Use Message Attribute Type to Receive Multiple File Types
This example shows how to use an attribute, which has the attribute type 'Message', to receive a file from the end user. The example uses the Code element to identify whether the file type is an image or a document.
Dialog
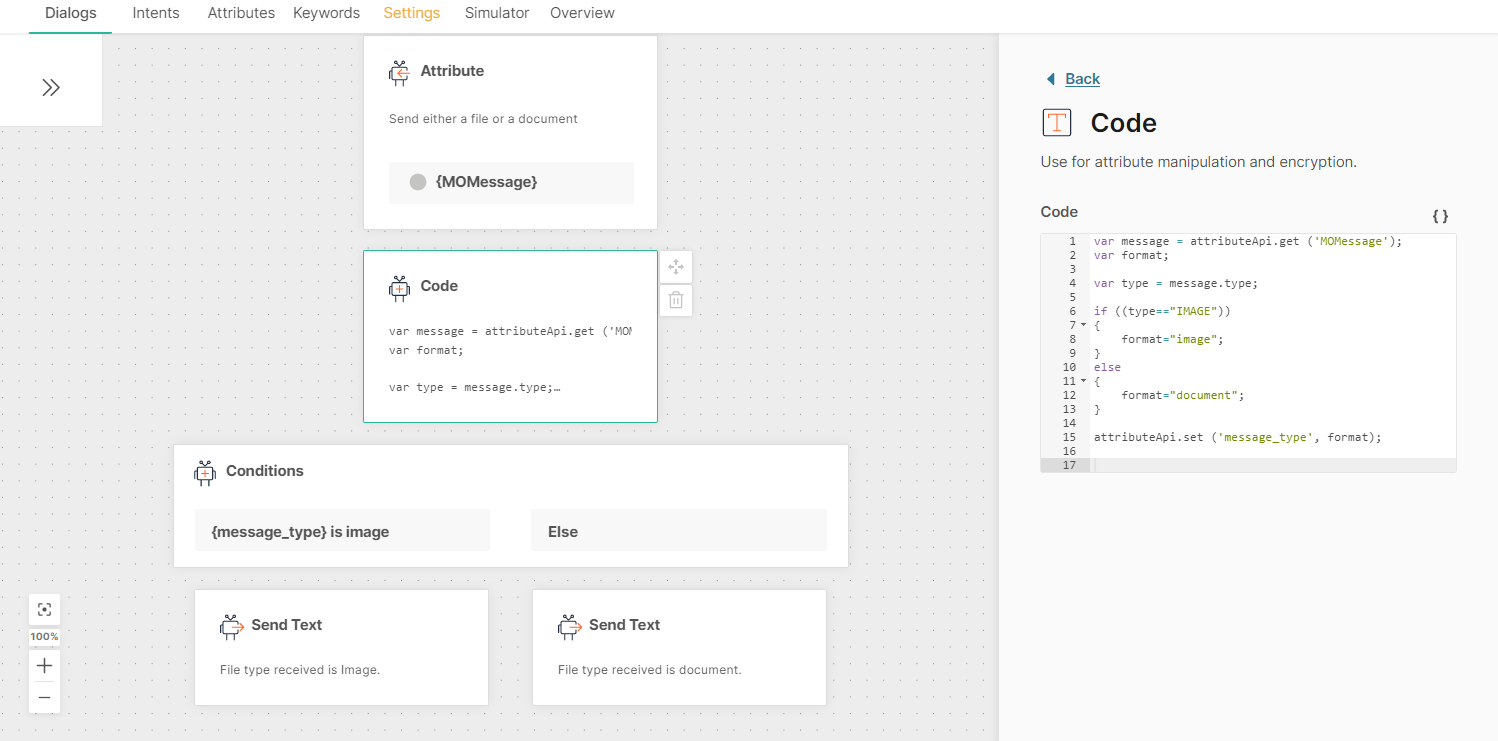
Code
var message = attributeApi.get ('MOMessage');
var format;
var type = message.type;
if ((type=="IMAGE"))
{
format="image";
}
else
{
format="document";
}
attributeApi.set ('message_type', format);
where
- MOMessage is of attribute type Message
- message_type is of attribute type Text
Explanation of the Dialog and Code
-
The MOMessage attribute stores the file sent by the end user.
-
The Code element does the following:
- var message = attributeApi.get ('MOMessage');
Uses the 'attributeApi' object to get the value of the MOMessage attribute - var type = message.type
Uses the 'type' property to extract the file type, and assigns the value to the variable, type - Uses the if-else statement to identify whether the 'type' variable is 'image' or 'document'. If it is image, assigns the value 'image' to the variable, format. Else, assigns the value 'document' to the variable, format
- attributeApi.set ('message_type', format);
Uses the 'attributeApi' object to set the value of the 'format' variable to the attribute, message_type
- var message = attributeApi.get ('MOMessage');
-
The Conditions element branches the dialog depending on the value of the 'format' variable.
For WhatsApp messages, the code in this example works only if the end user uses the correct icon in WhatsApp to send the file. If the end user uses the Image icon in WhatsApp to send an image, the received file is recognized as an image. But if the end user uses the Attachment icon in WhatsApp to send an image, the received file is not recognized as an image.
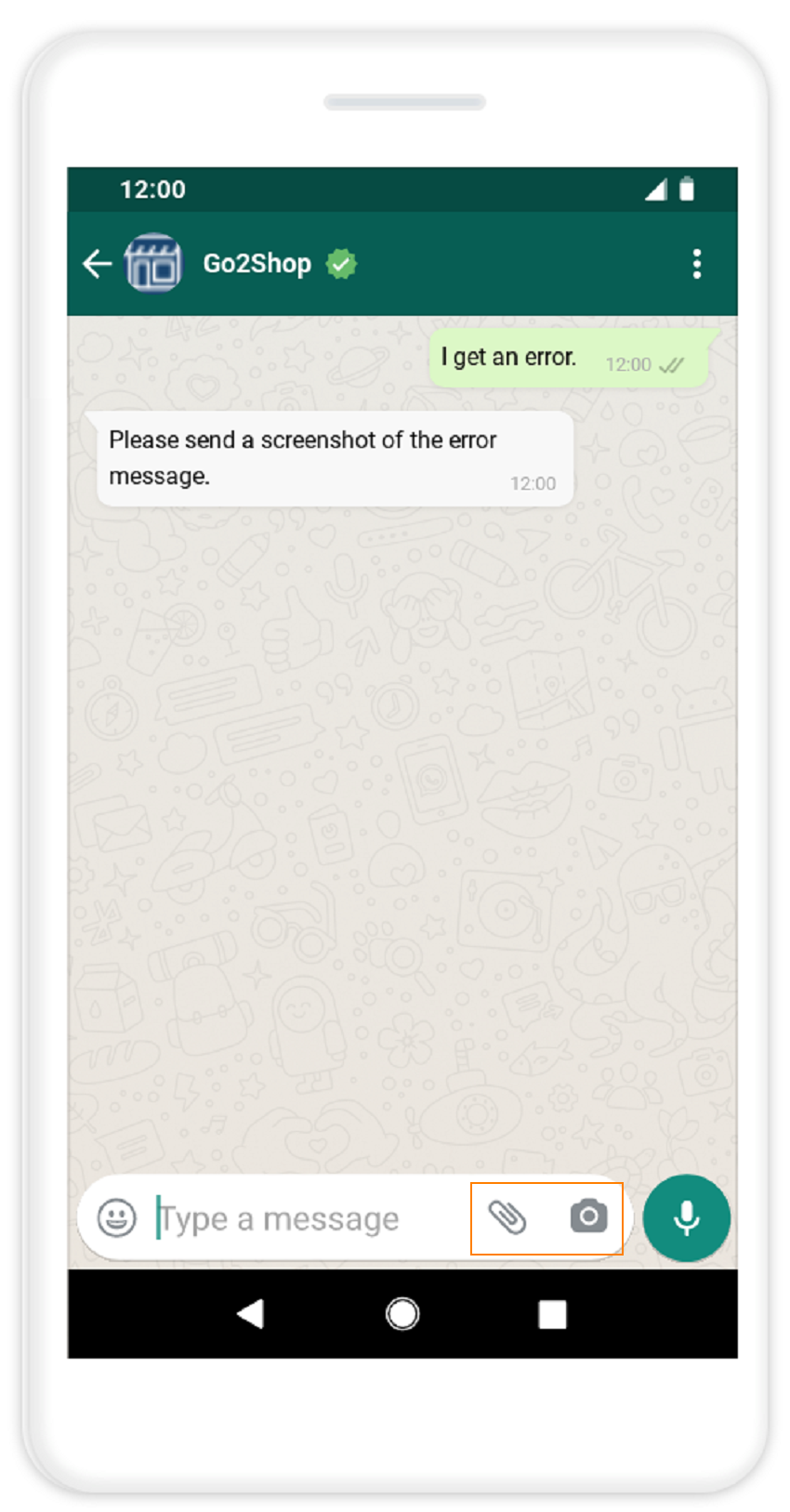
Change Date Format
A standard attribute that has the attribute type, date, stores the date in the format YYYY-MM-DD. If end users send the date in a different format, it is treated as an invalid input.
This example shows how to use the Code element to save the date in the format DD-MM-YYYY.
Set up Attributes
Create attributes and configure the fields as shown in the following image.
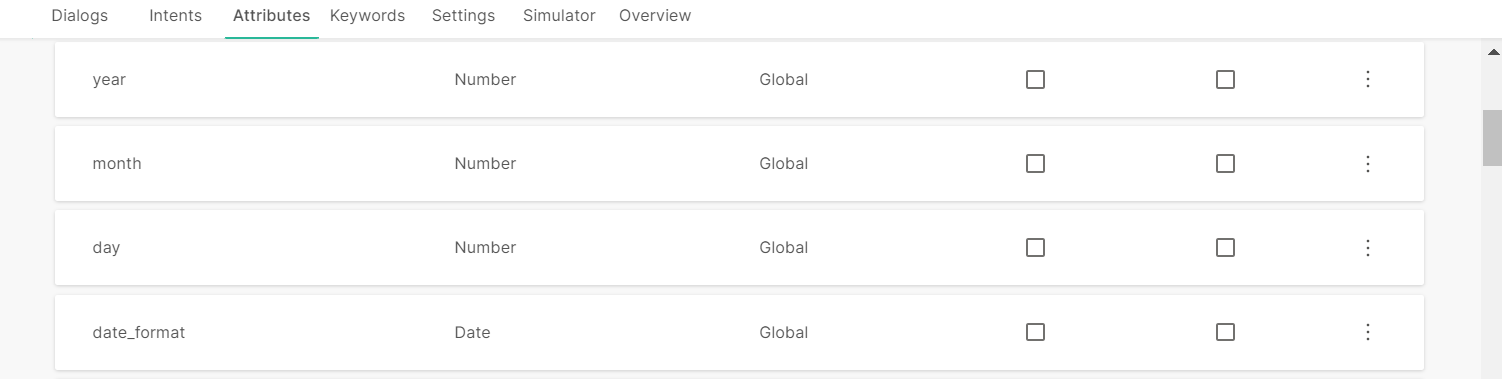
Code
const d = new Date(attributeApi.get('date'));
var day=d.getDate();
const m = new Date(attributeApi.get('date'));
var month=m.getMonth()+1;
const y = new Date(attributeApi.get('date'));
year = y.getFullYear();
if (day < 10) {
day = '0' + day;
}
if (month < 10) {
month = '0' + month;
}
var form= day +'-' + month + '-' + year;
attributeApi.set('day',day);
attributeApi.set('month', month);
attributeApi.set('year', year);
attributeApi.set('date_format', form);
Dialog
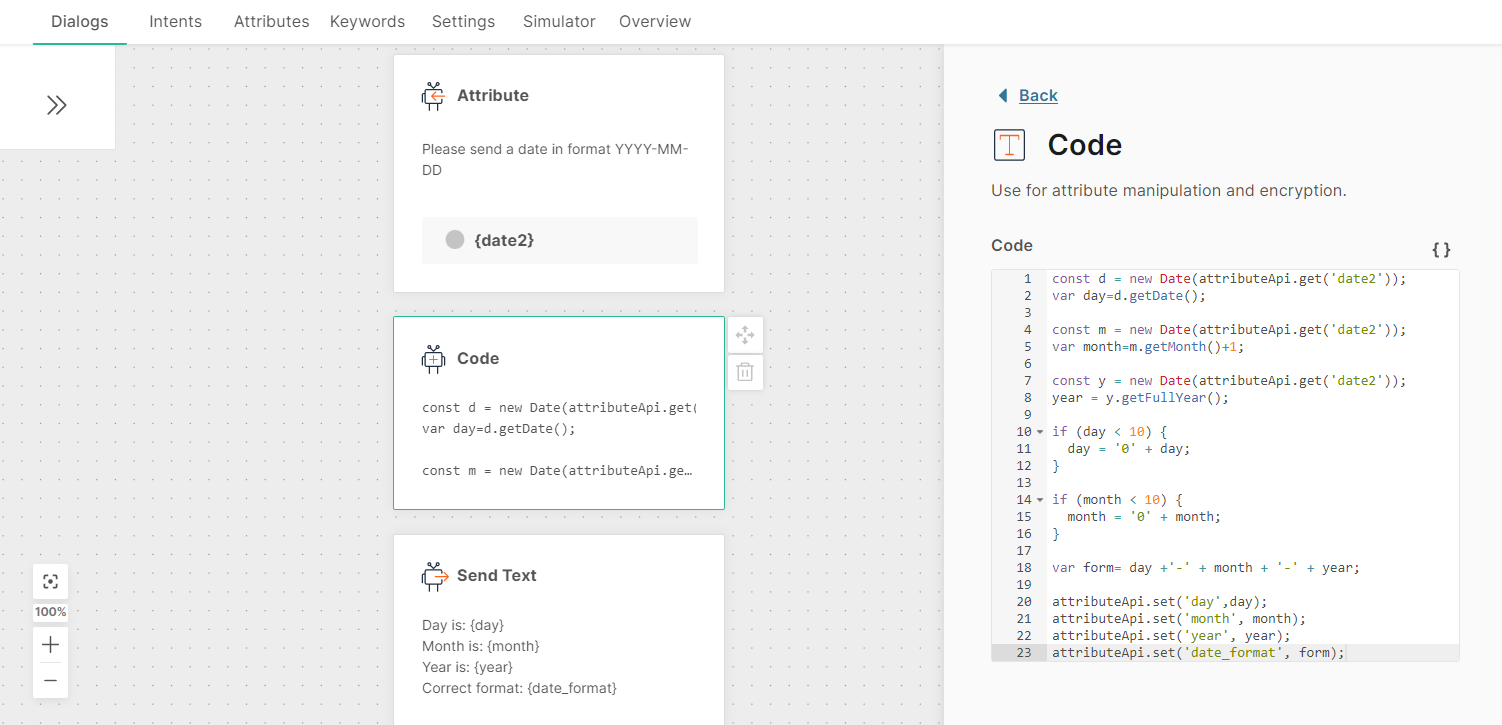
Simulator
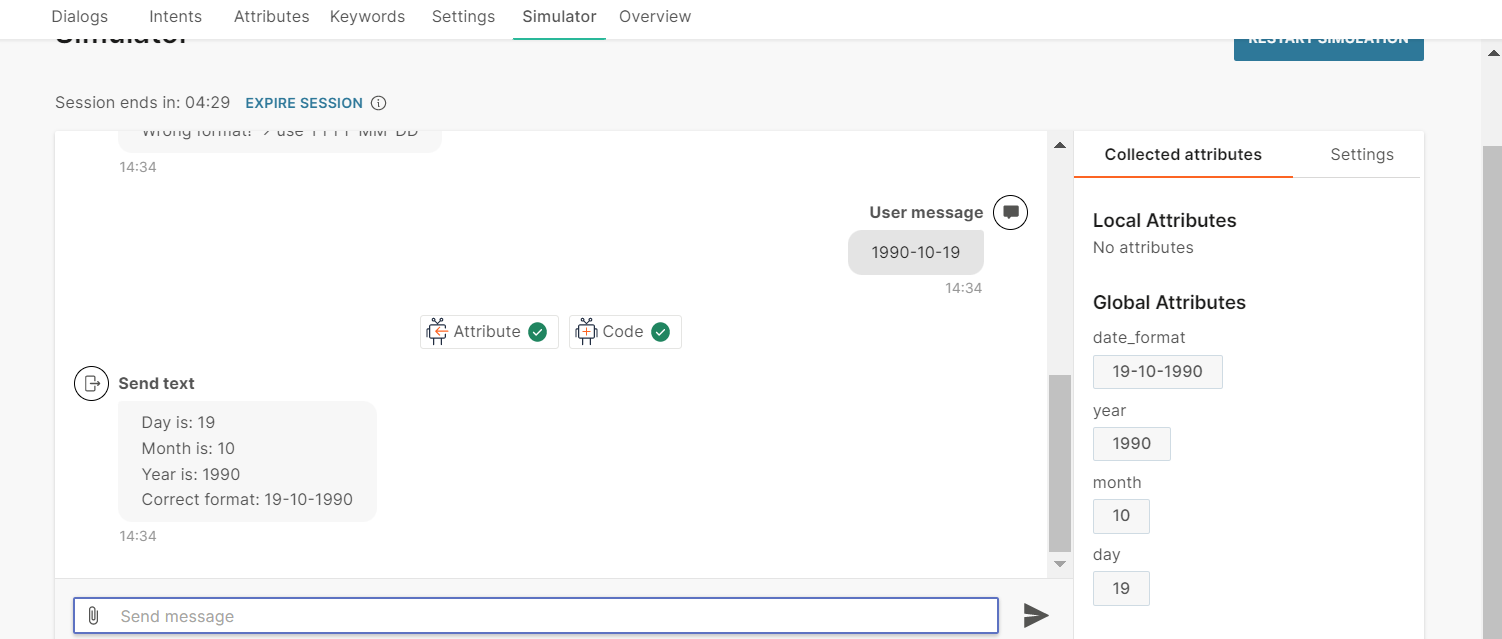
Change Phone Number Format
If end users send you a phone number in an incorrect format, you can convert it to the required format. This example shows how to do the following:
- If the number starts with the country code, there is no change.
- If the number starts with 0, it remove the 0 and add the country code.
- If the number does not start with either the country code or 0, add the country code.
This example uses the country code 63.
Code
var a= attributeApi.get('mobile_number');
if (a.startsWith("639")) {
attributeApi.set('new_number', a);
}
else if (a.startsWith("0")) {
var b="63" + a.substring(1);
attributeApi.set('new_number', b);
}
else if (a.startsWith("9")) {
var b="63" + a;
attributeApi.set('new_number', b);
}
Dialog
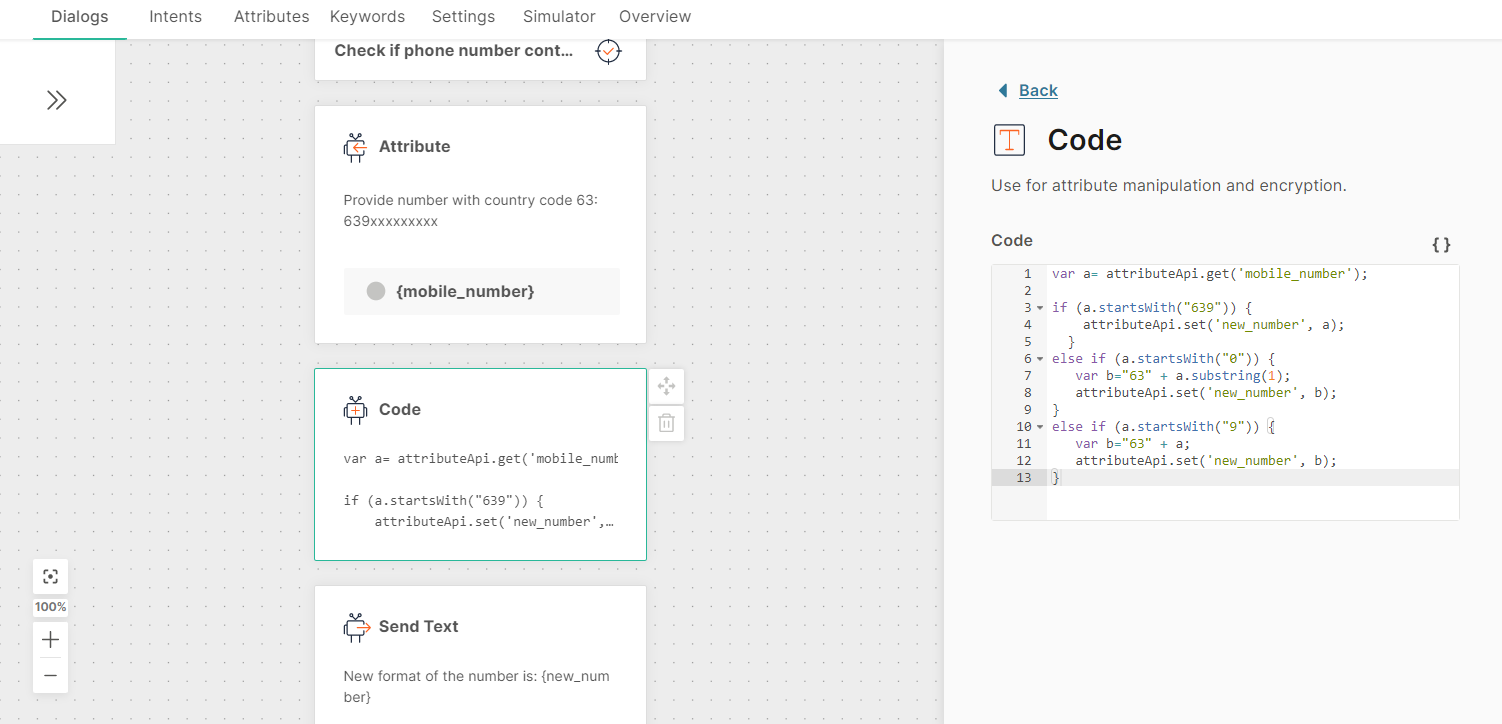
Find the nearest location
You can calculate the location of a service that is nearest to the end user. Example: Location of the nearest ATM.
Set up Attributes
Create attributes and configure the fields as shown in the following image.
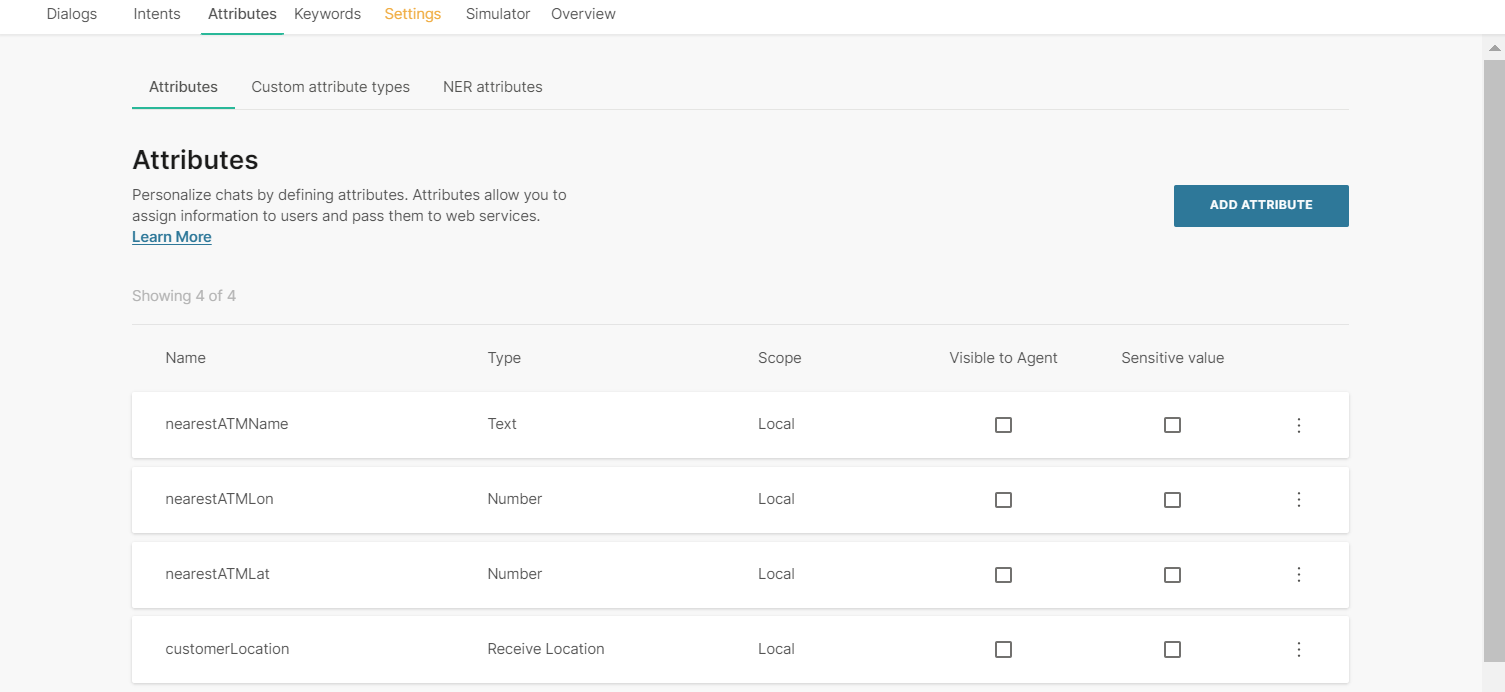
Code
var tempDataList = [
{'lat': -23.607171362032318, 'lon': -46.69675437970512, 'name': 'São Paulo'},
{'lat': -25.423777049024135, 'lon': -49.26882764791356, 'name': 'Curitiba'},
];
var locationClient = attributeApi.get('customerLocation');
var locationCode = {'lat': locationClient.latitude, 'lon': locationClient.longitude};
var i=1;
function distance(lat1, lon1, lat2, lon2) {
var radlat1 = Math.PI * lat1/180;
var radlat2 = Math.PI * lat2/180;
var theta = lon1 - lon2;
var radtheta = Math.PI * theta/180;
var minimalDistance = Math.sin(radlat1) * Math.sin(radlat2) + Math.cos(radlat1) * Math.cos(radlat2) * Math.cos(radtheta);
if (minimalDistance /> 1) {
minimalDistance = 1;
}
minimalDistance = Math.acos(minimalDistance);
minimalDistance = minimalDistance * 180/Math.PI;
minimalDistance = minimalDistance * 60 * 1.1515;
minimalDistance = minimalDistance * 1.609344;
return minimalDistance;
}
var minimalDistance = [];
tempDataList.forEach(myFunction);
function myFunction(value, index, array){
minimalDistance[index] = distance(locationCode.lat, locationCode.lon, value.lat, value.lon);
}
var closestDistance = Math.min.apply(null , minimalDistance);
var index = minimalDistance.indexOf(closestDistance);
attributeApi.set('nearestATMLat', tempDataList[index].lat);
attributeApi.set('nearestATMLon', tempDataList[index].lon);
attributeApi.set('nearestATMName', tempDataList[index].name);
Dialog
The following is an example of the dialog.
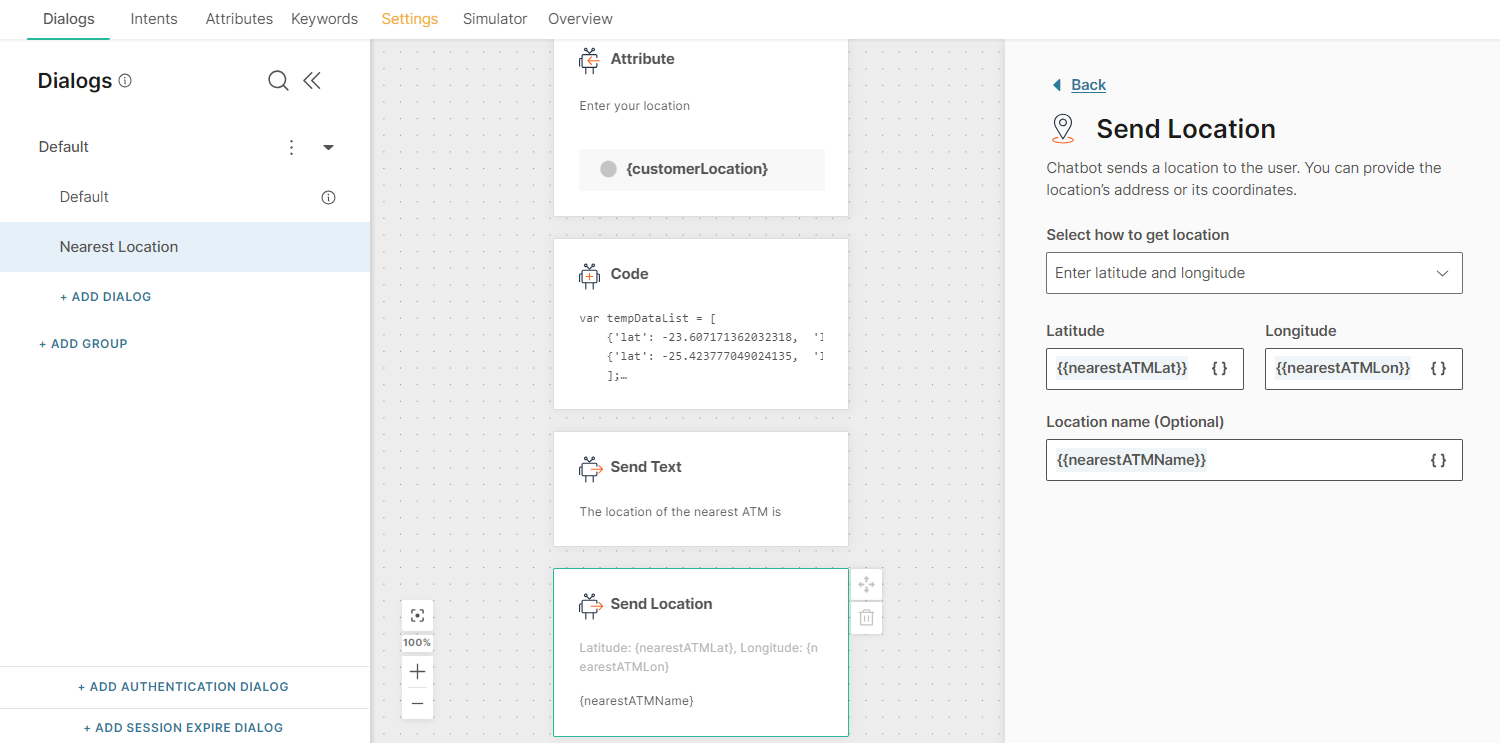
Simulator
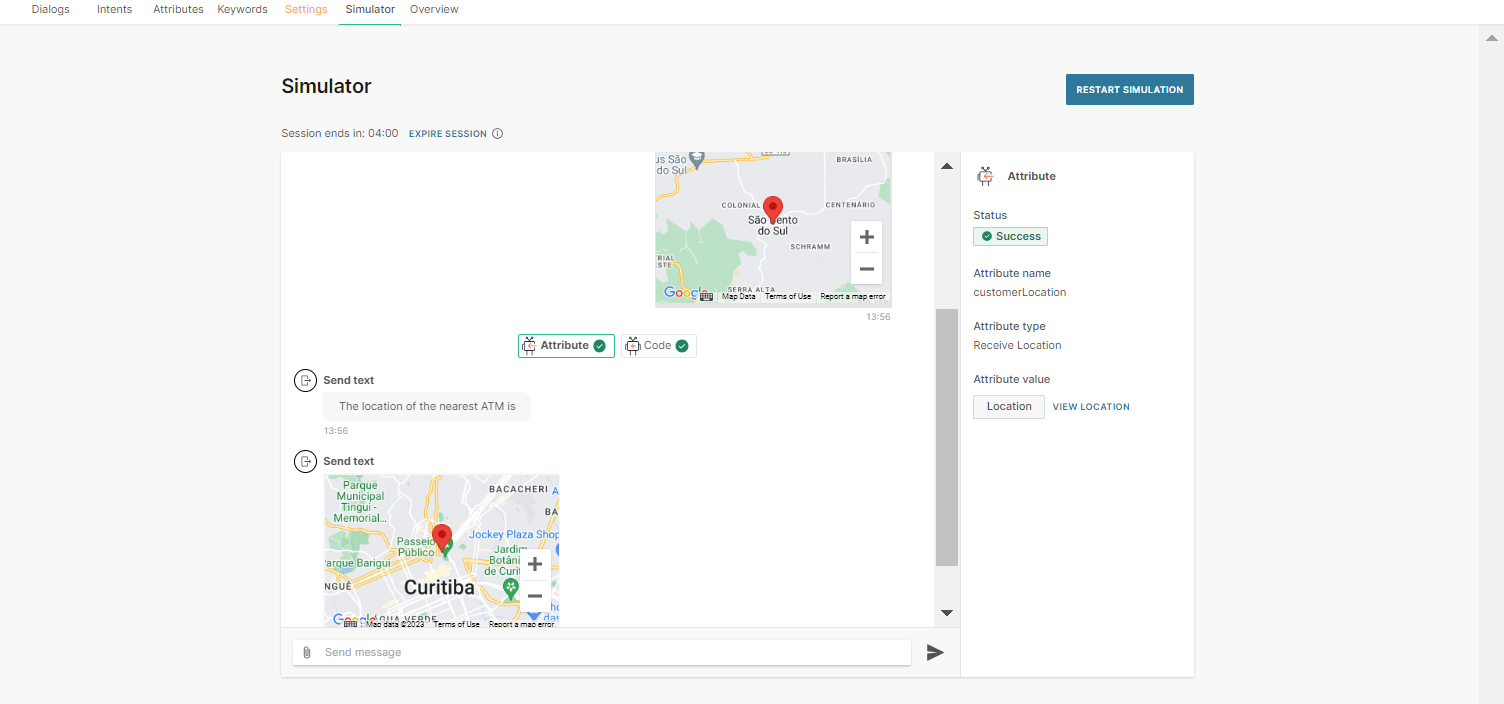