Blog
Develop and grow customer relationships over any channel, any device, anywhere. Powered by Infobip full-stack cloud communication platform
Results for
results found
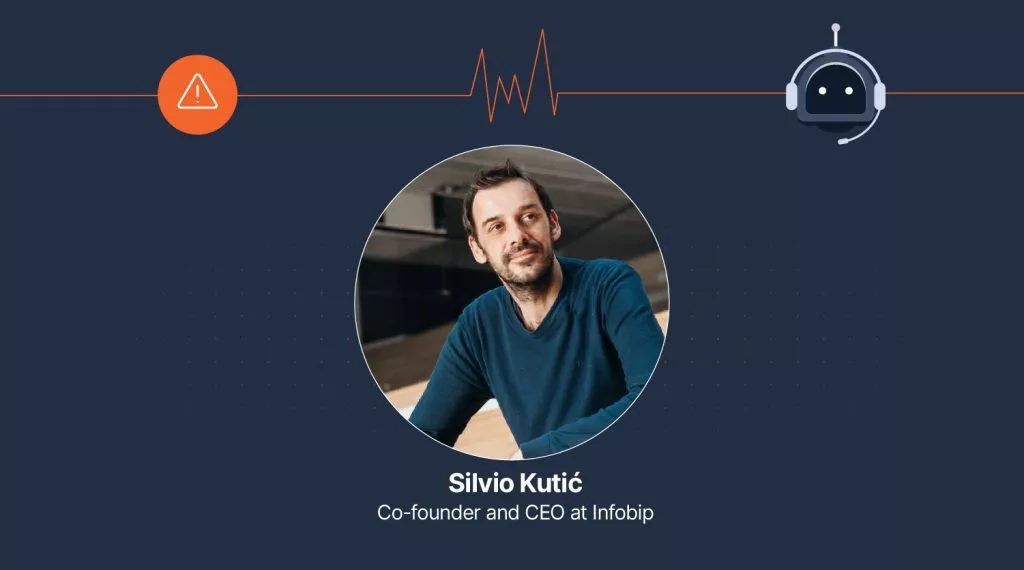
How robocalls are threatening election integrity
Robocalls have become a major threat to election integrity, spreading misinformation and potentially influencing voters. Find out what steps can be taken to stop scammers and protect our democracy.
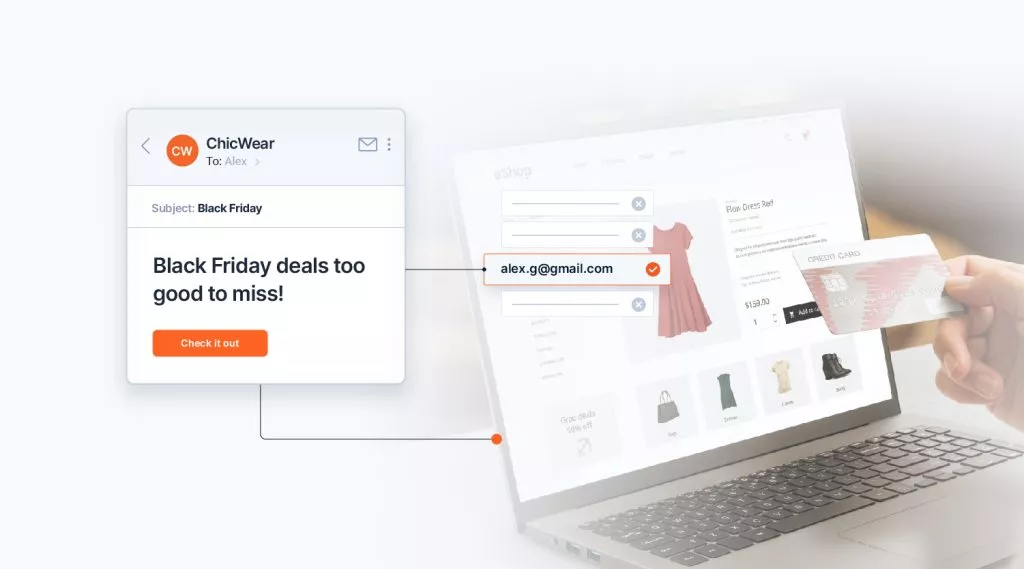
How to nail your email delivery strategy ahead of Black Friday and the shopping season
With the peak shopping season now extending from October to January, now is the time to ensure that your email strategy and infrastructure is ready to capitalize. Find out how with our detailed guide.
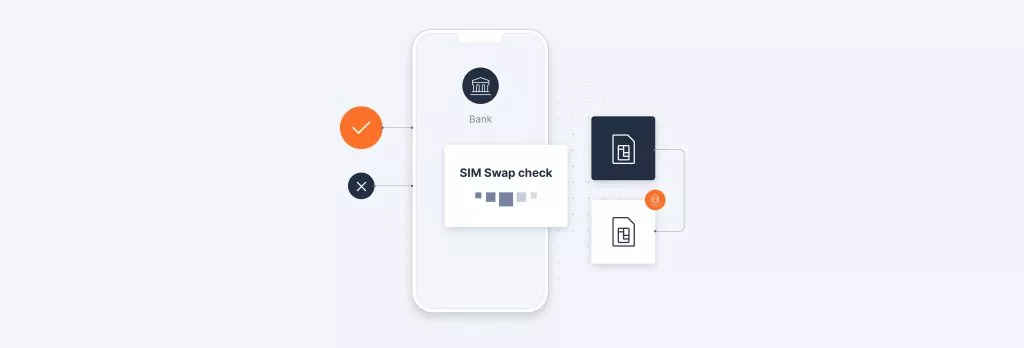
SIM swapping fraud: What is it and how to prevent it
Learn about the dangers of SIM swapping fraud, and what can be done to prevent it from causing serious financial loss.
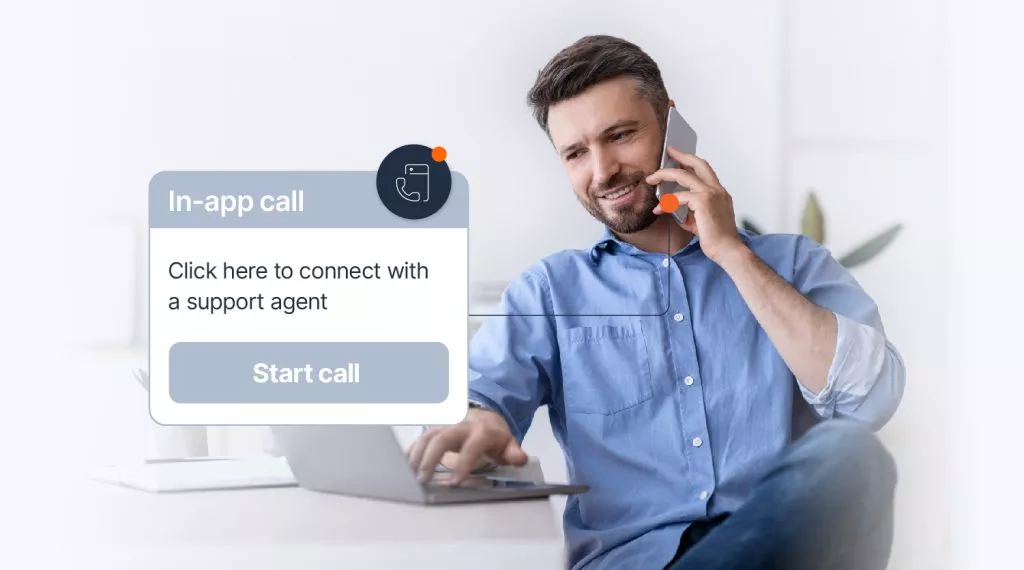
All about the rising trend of in-app voice calls
Learn how to incorporate voice calls into your app or platform to offer customers seamless and convenient customer support.
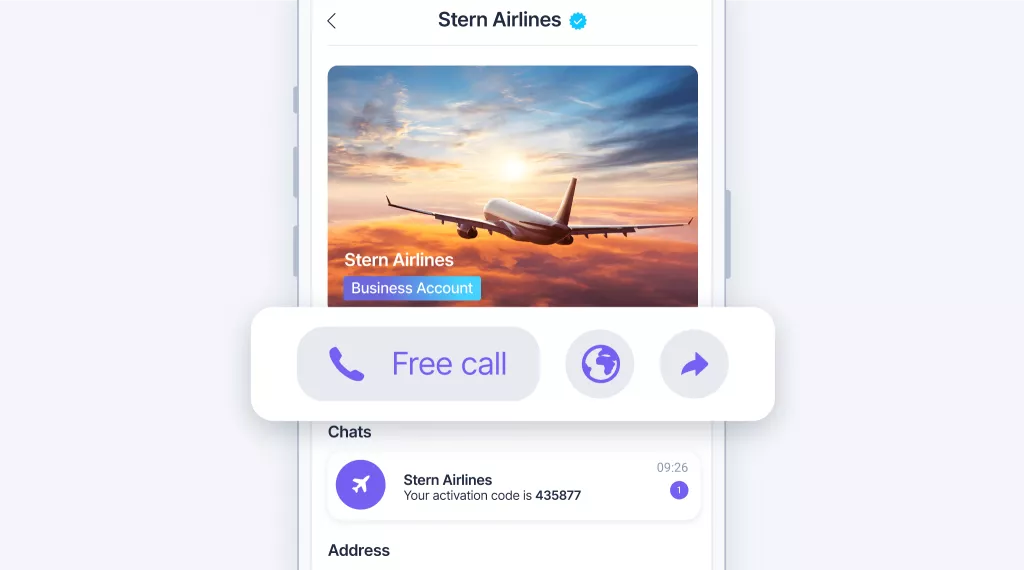
Transform your business communication with Viber Business Calls
Improve customer satisfaction with Viber Business Calls. Find out how to provide exceptional service and personalized interactions.
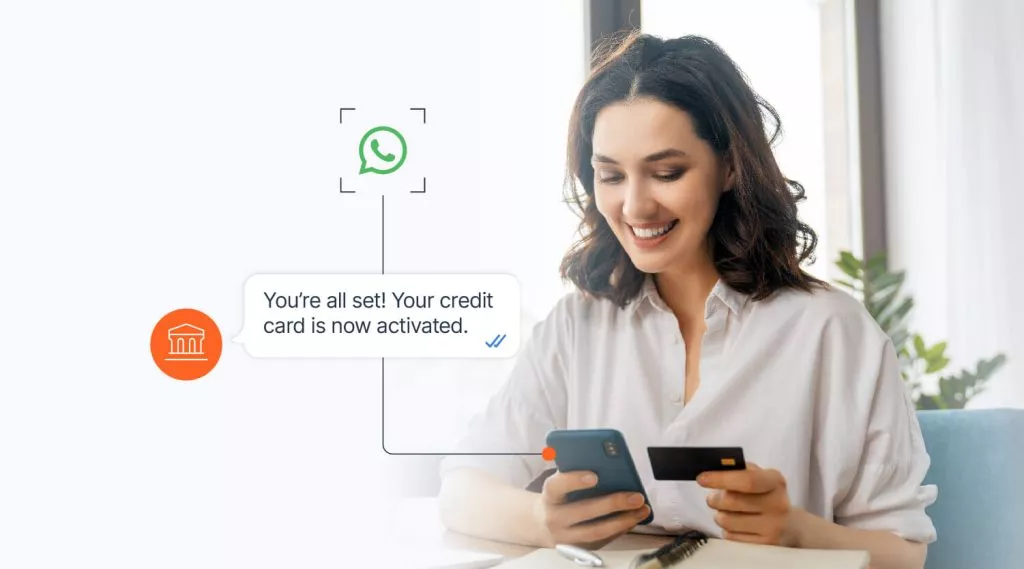
WhatsApp banking: 19 examples for better customer experiences
Deep dive into the world of banking via WhatsApp and learn about benefits, best practices, use cases, and how to get started.
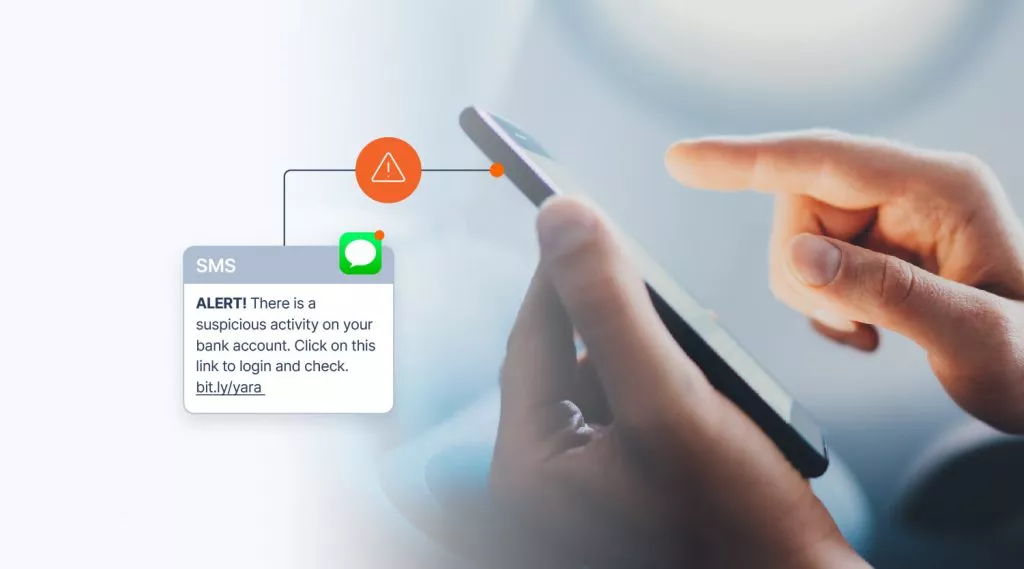
What is smishing and how to prevent it
Read about smishing, one of the biggest threats to the mobile industry, and learn what mobile operators, brands, and users can do to protect themselves.
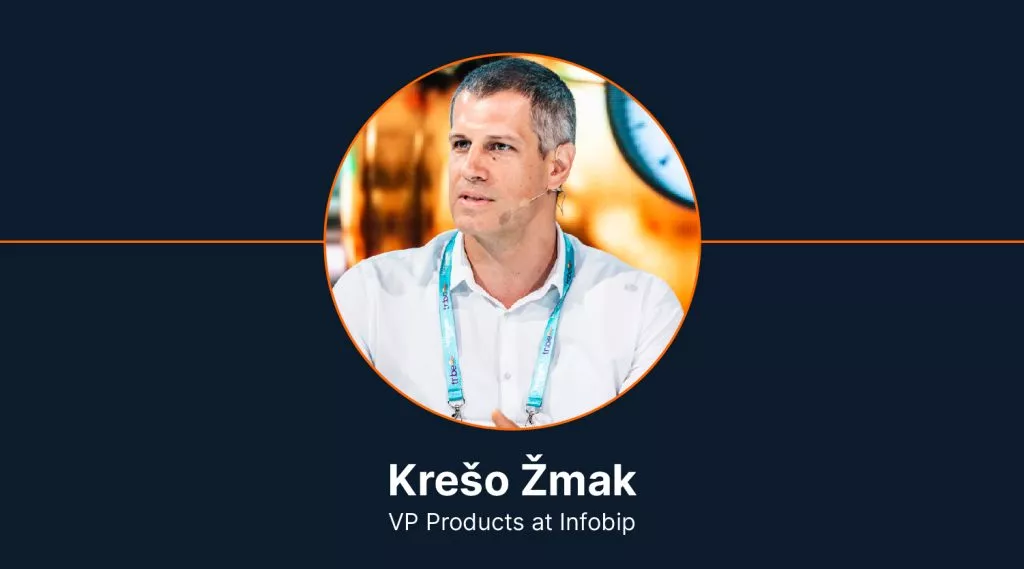
Top trends for the future of B2C communication
Find out what technologies will power the future of business-to-consumer messaging, and how our solutions are built to help you adapt to these changes in customer communication.
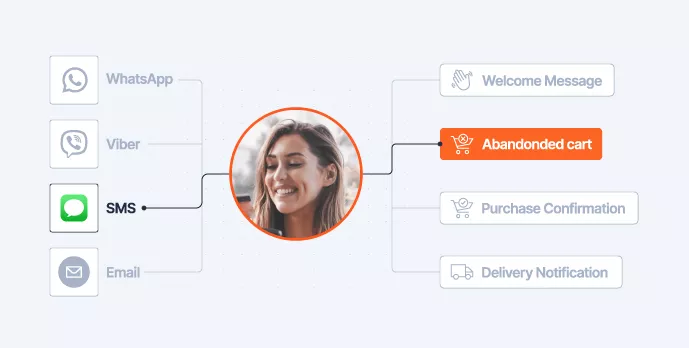
Omnichannel retail strategy for transformed customer experiences
Transform customer experiences with this omnichannel retail strategy for improved customer journeys and a smoother path to purchase.
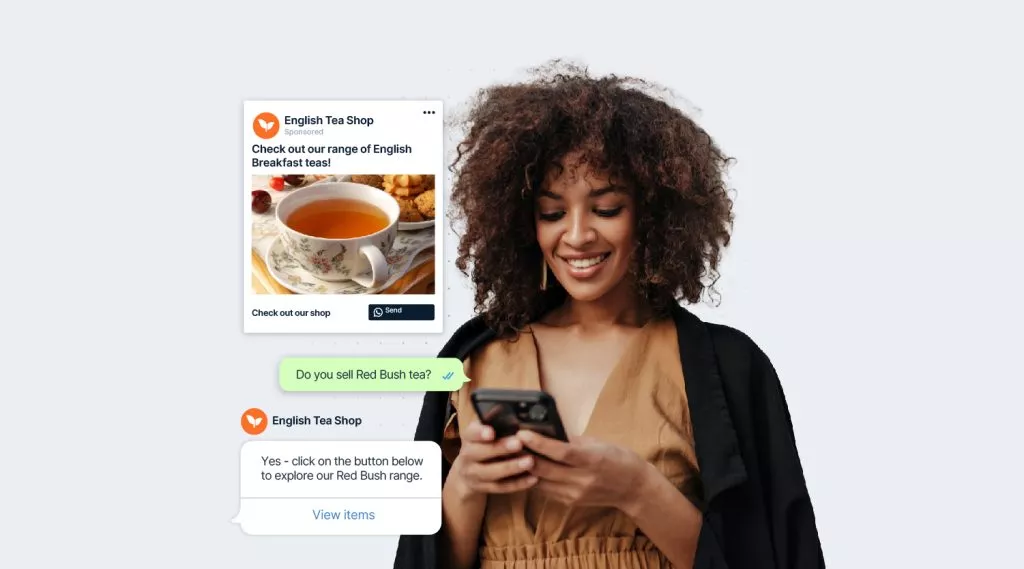
WhatsApp marketing: Best practices and strategies for 2024
How to craft an effective WhatsApp marketing strategy that incorporates the apps latest features and tools designed especially for business.
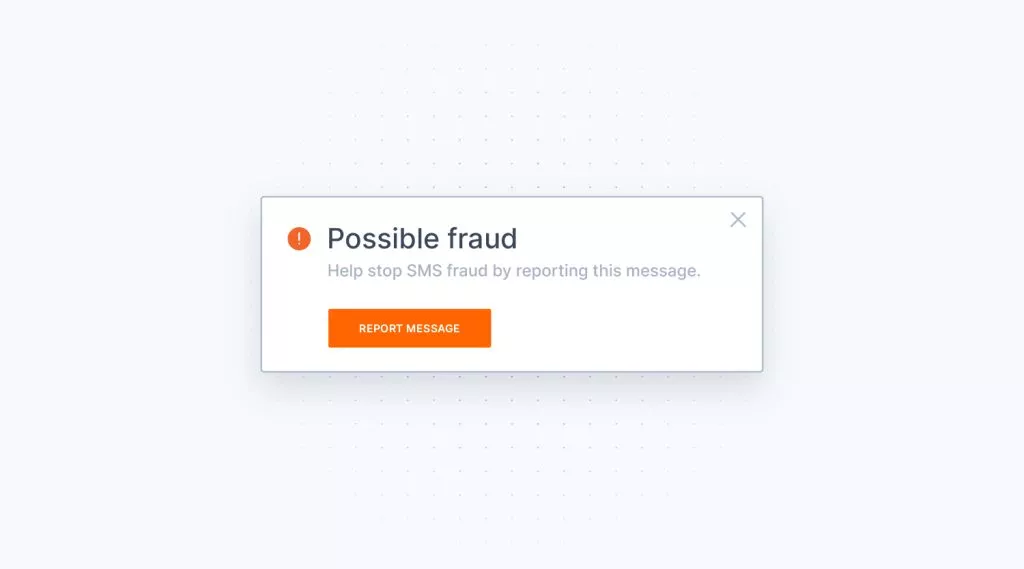
A complete guide to SMS fraud detection and prevention
Learn about the latest SMS fraud tactics criminals are using, how they work, and how they can be prevented.
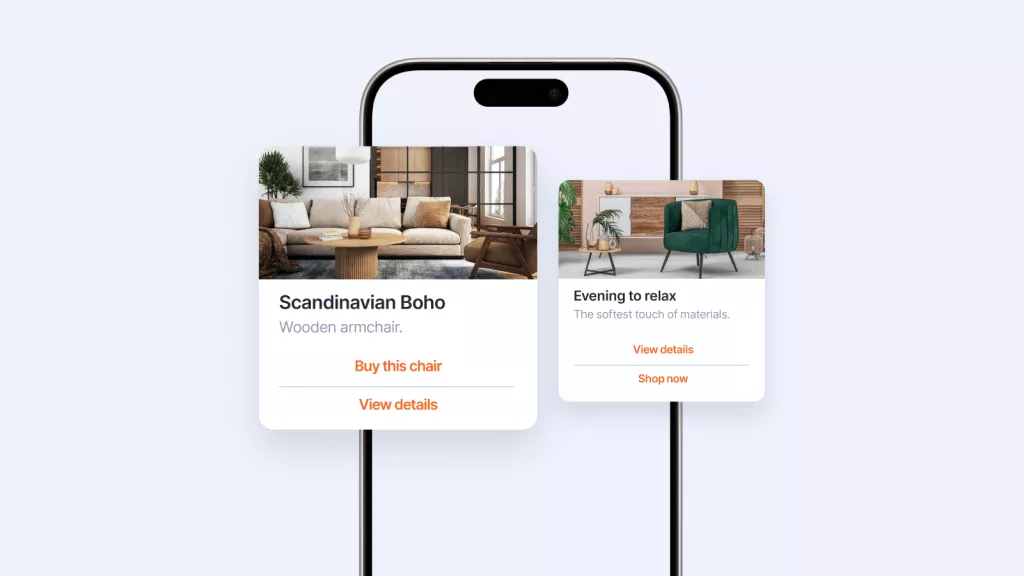
Apple to support RCS in 2024: Everything we know so far
Apple announced it will support RCS on iOS devices in 2024. Find out what it could mean for the future of messaging.
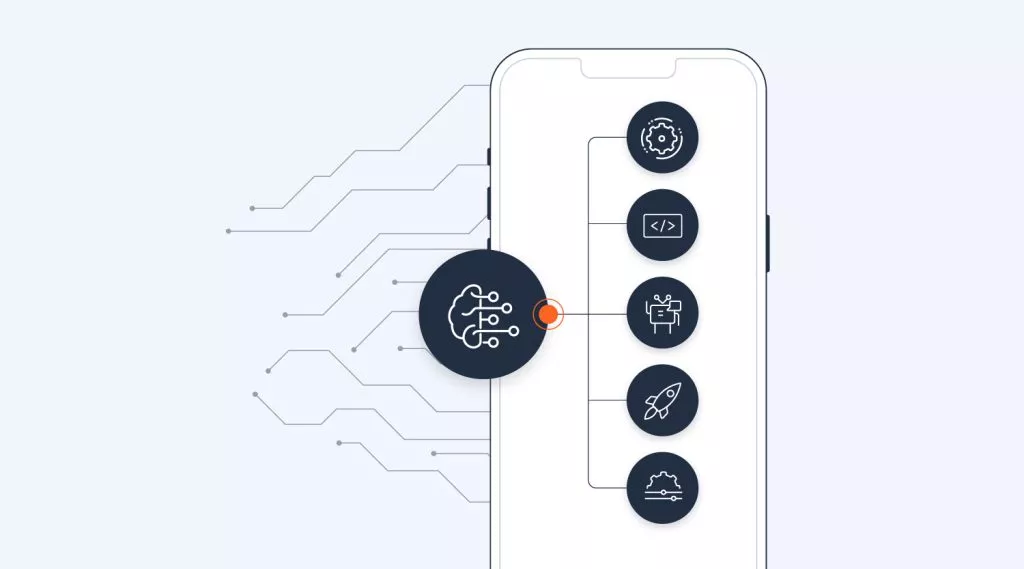
Understanding large language models vs. generative AI
Uncover the differences between large language models and generative AI and how these tools can be leveraged by businesses.
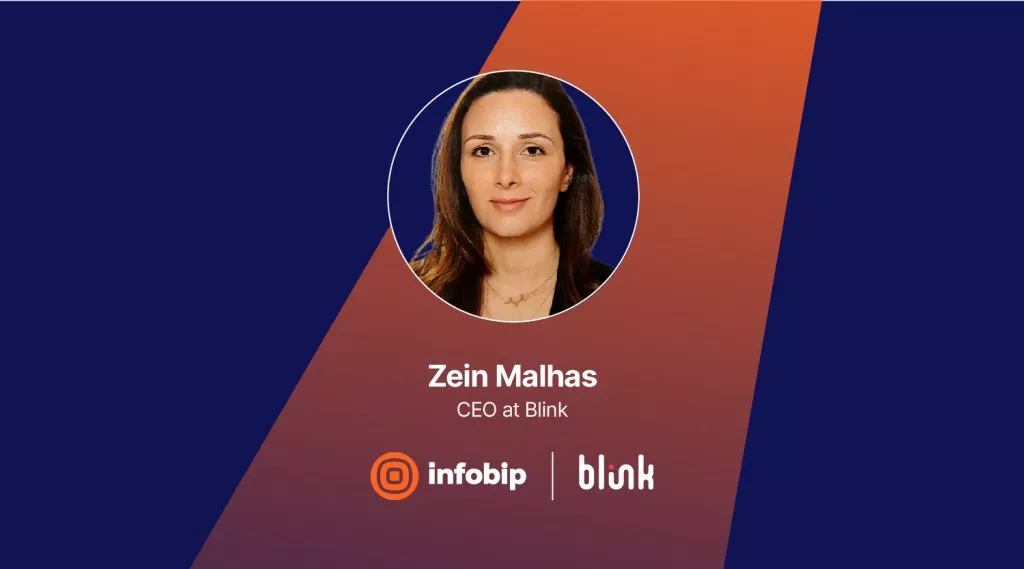
Blink: How AI-powered neobanks are changing the game for banking
Learn how Blink, an AI-powered neobank is transforming banking and setting a new standard for customer satisfaction and convenience.
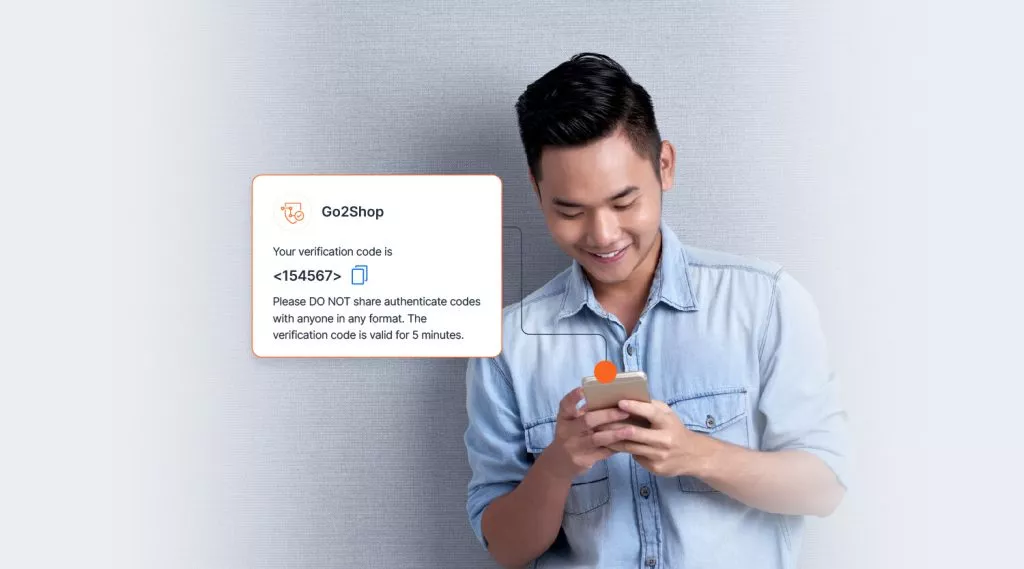
Zalo for business: The complete guide
Discover Zalo, Vietnam’s top messaging app, and learn how it can enhance your business’ communication in the Vietnamese market.
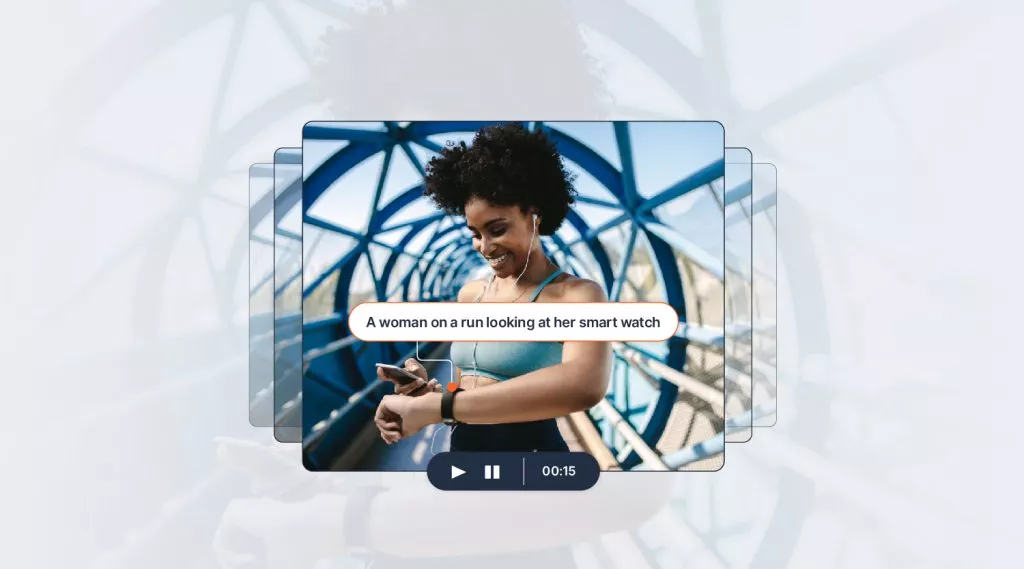
Sora: All about text-to-video AI and what it could mean for businesses
Discover about OpenAI’s text-to-video model, Sora, and what potential it holds for improving customer experiences.
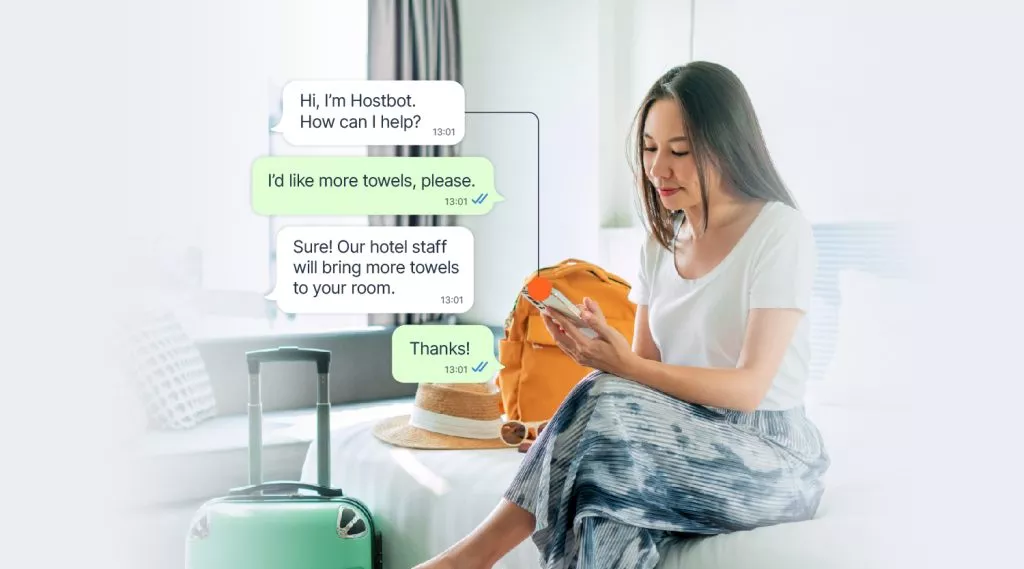
Why your hotel needs a chatbot
Learn how artificial intelligence is disrupting the hospitality industry and how chatbots can help hotels exceed customer expectations while lowering costs.
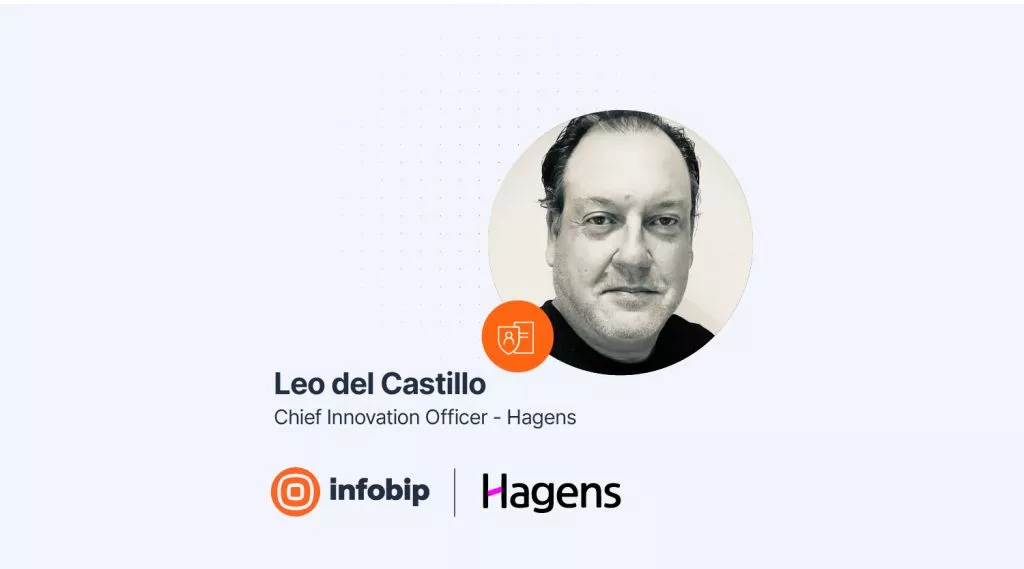
From clicks to conversations: How Hagens is driving retail success with conversational marketing
Dive into the world of conversational marketing in the retail sector with Hagens. See how they are leveraging this strategy to boost conversions and build meaningful relationships with customers.
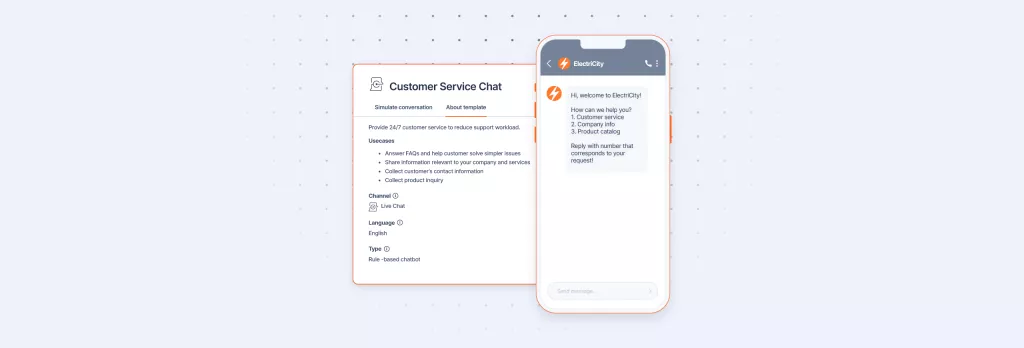
Free chatbot templates: Ready to launch in minutes
Read on to learn more about chatbot templates: benefits, usage, and best practices for seamless integration.
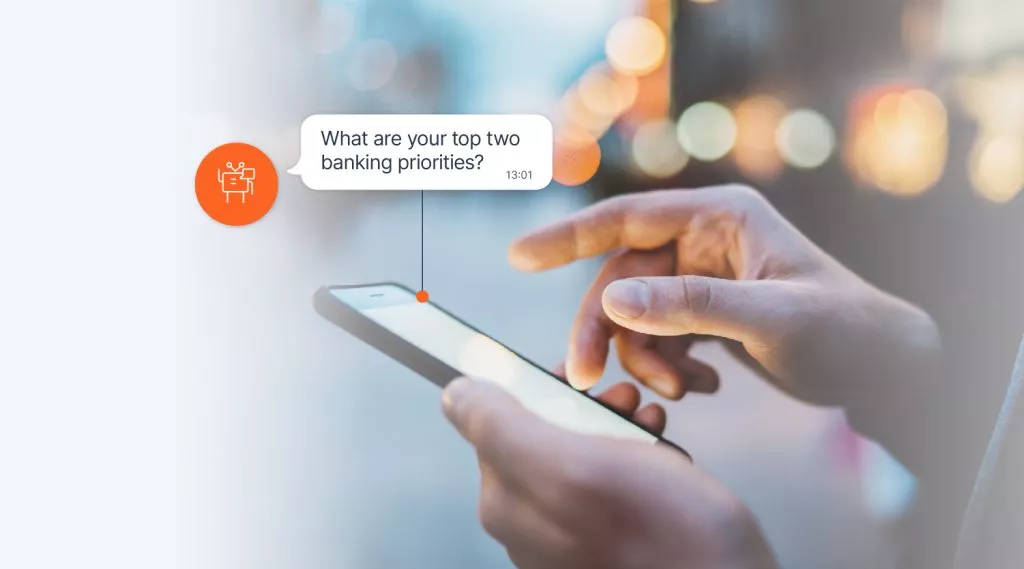
From hype to hero: How chatbots can revolutionize your bank’s customer experience
Dive into practical applications of banking chatbots, voice-activated solutions, and generative AI that are already transforming banking experiences.
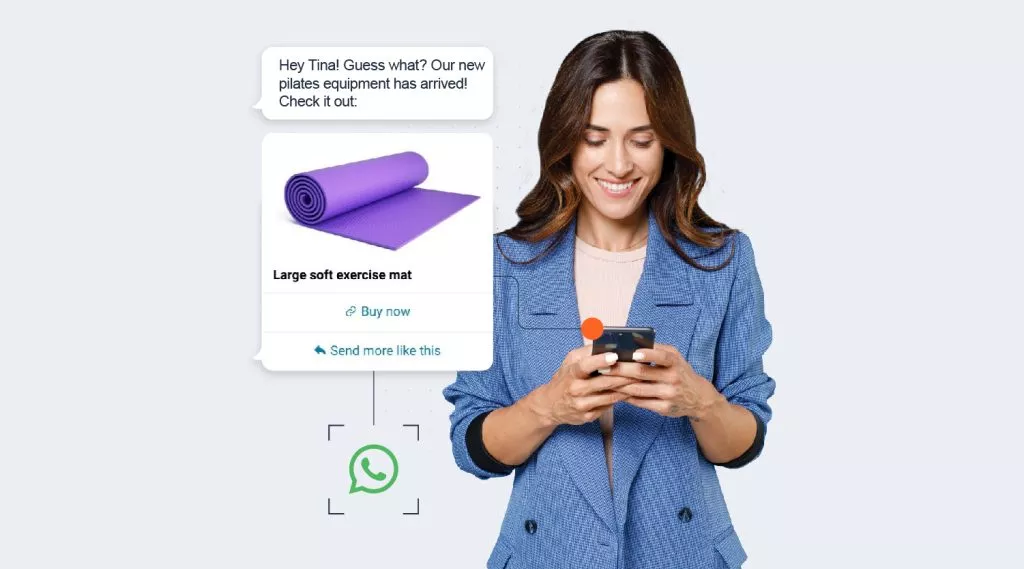
WhatsApp marketing messages: How to build stronger customer connections with Meta’s new limits
Meta has introduced new limits to the number of marketing messages businesses can send to a customer. Find out how the right communication tools can turn these new limits into an opportunity instead of a threat for your business.
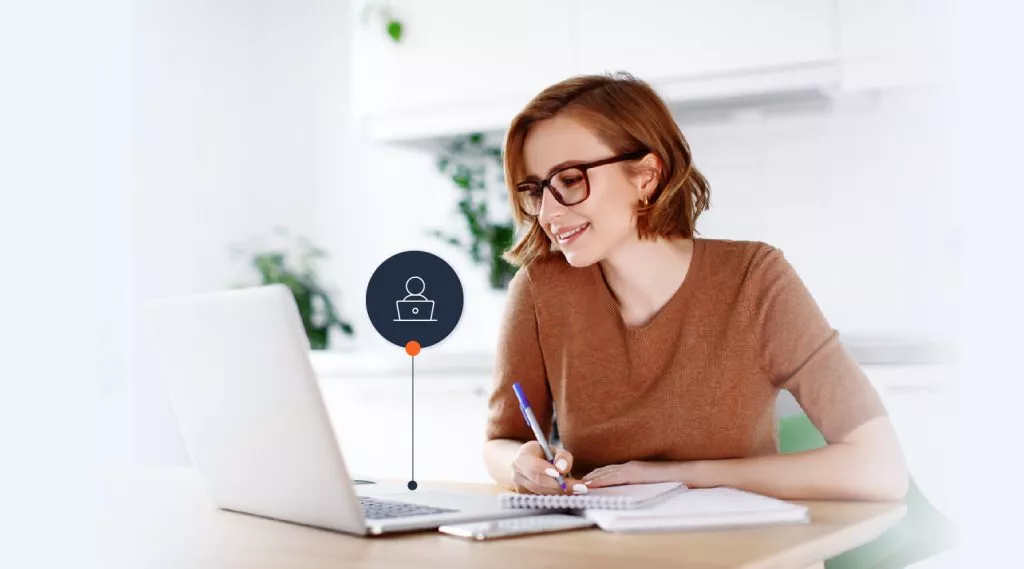
19 of the best free AI courses you can start today
Level up your AI and machine learning knowledge in 2024 with our curated list of the best free courses and resources available on the web.
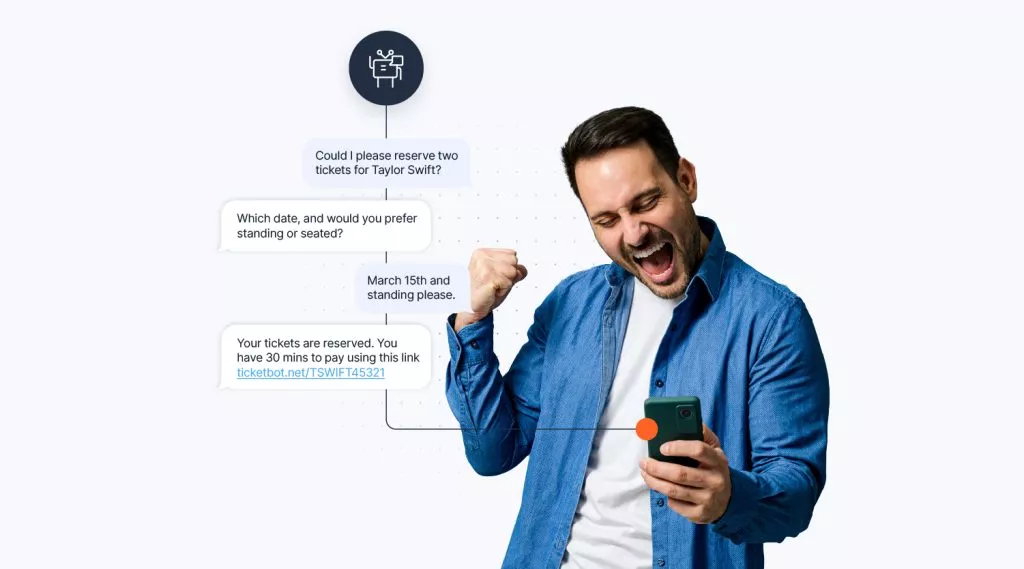
How to design and build an SMS chatbot
Everything you need to know about SMS chatbots, including what makes them so useful, and a step-by-step guide to building one.
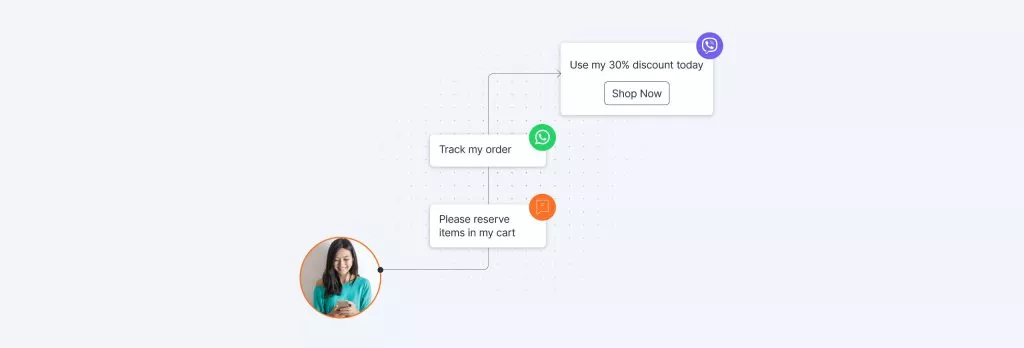
The retailer’s guide to customer engagement
Learn everything you need to know about customer engagement and how retailers can drive success a digital world.
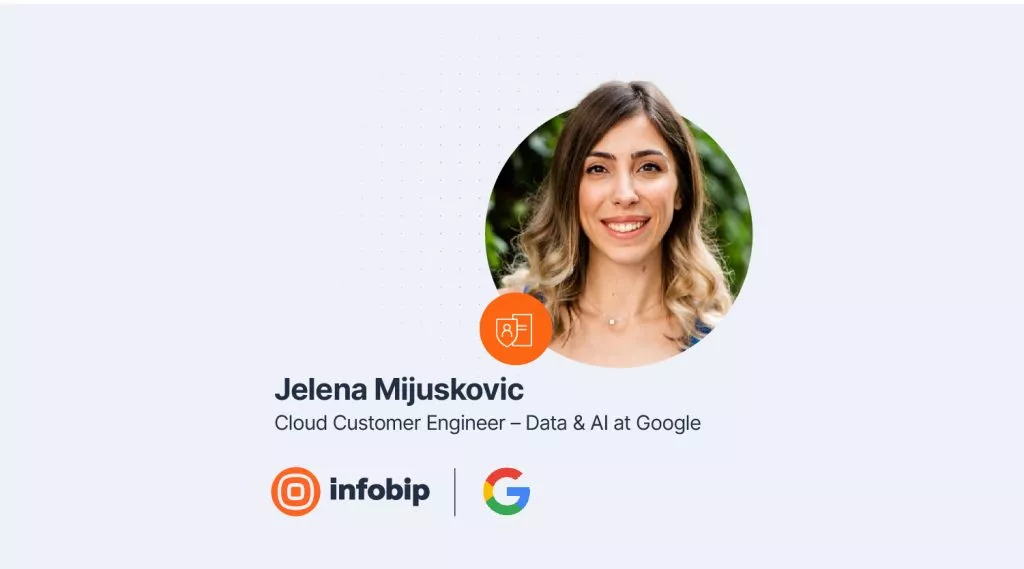
Tips and tricks for a successful GenAI strategy
Discover the latest tips and tricks for implementing a successful GenAI strategy, straight from Google’s Data and AI Engineer, Jelena Mijuskovic.
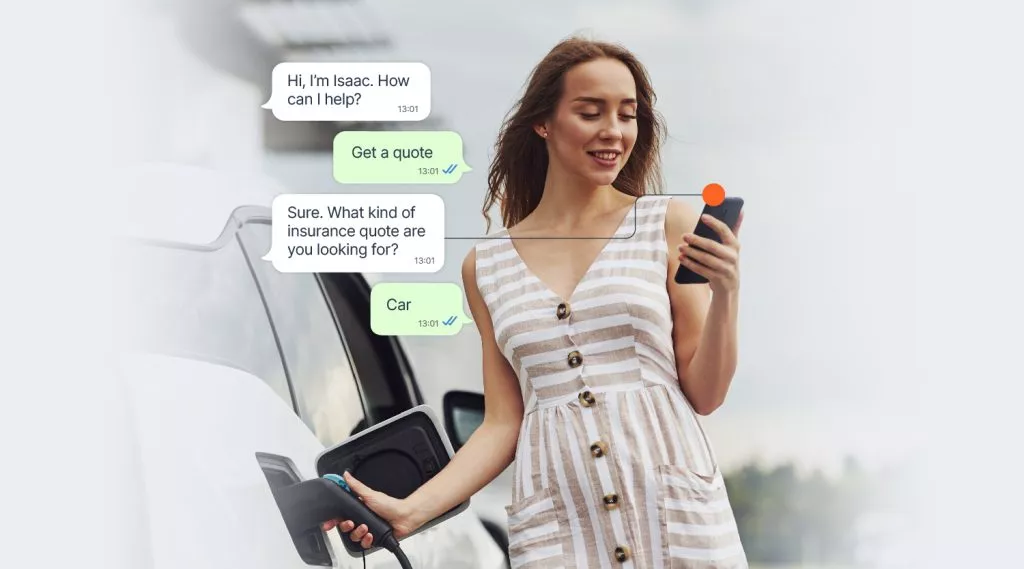
Beyond Automation: How insurance chatbots can provide a conversational experience
Conversational and generative AI are set to change the insurance industry. Read about how using an AI chatbot can shape conversational customer experiences for insurance companies and scale their marketing, sales, and support.
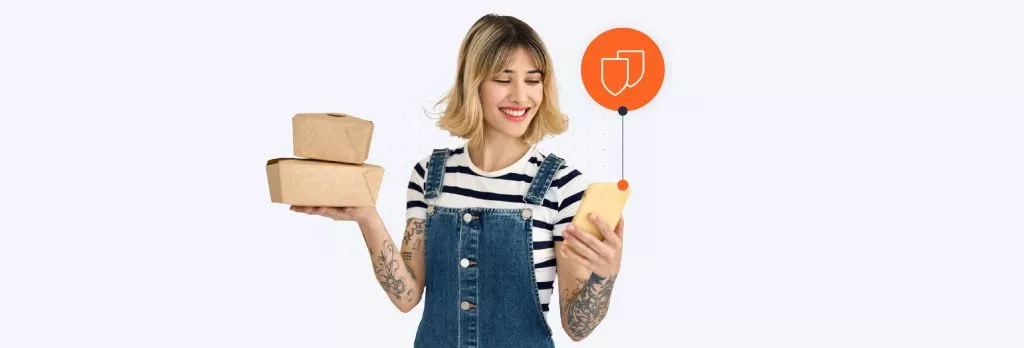
Securing the ride-sharing and food delivery journey for customers and drivers with RCS Business Messaging and authentication
Find out the best ways to offer a secure customer journey for on-demand ride sharing and food delivery services.